|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
Quests::Q&A This is the quest support section |

01-29-2009, 09:14 PM
|
Sarnak
|
|
Join Date: Jul 2005
Location: Ohio
Posts: 72
|
|
Raid Mob Spawns Adds
So I am trying to get a raid mob to spawn adds at certain amounts of HP. It breaks down like this:
75% = 3 lvl 68 adds
50% = 5 lvl 66 adds
25% = 8 lvl 65 adds
Now, I'm not too good at writing quests, so I was using GeorgeS's quest program as a template for this.
Code:
sub EVENT_COMBAT {
if ($combat_state == 1) {
quest::setnexthpevent(75);
}
}
sub EVENT_HP {
if ($hpevent <= 75) {
quest::spawn(999270)
}
}
This is just the 75% HP event, and if I can get this part working, the other parts will be ok. What I really need help on is the quest::spawn part. The 999270 is the first set of 3 mobs. Now, what am I supposed to be putting for the "grid" and "guildwarset"? I was thinking to just put 0 and then the x,y,z.
__________________
Building Server
Legends of Time - Full Custom/Legit Roleplay, need devs
|
 |
|
 |

01-29-2009, 09:54 PM
|
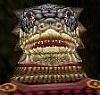 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Few things:
when making quests, make sure you end your commands with a semicolon ; and make your "if" statements have a { and a matching } when you are done with them. This is required and should become a habit.
Guildwarset and grid can be 0.
I'm not sure what guildwarset does (obsolete code?) but grid determines if the NPC is on a grid *AFTER* it spawns. This is useful for having adds roam a specific location.
You can look here for grid creating commands.
http://www.eqemulator.net/wiki/wikka...aypointEditing
In addition, if you want to make NPCs spawn on the boss, or maybe a random direction 20 meters from the boss, you can use math to implement this:
This will spawn an NPC on top of where the NPC that triggered it is.
Code:
sub EVENT_COMBAT {
if ($combat_state == 1) {
quest::setnexthpevent(75);
}
}
sub EVENT_HP {
if ($hpevent <= 75) {
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
}
At a direction random from where the NPC started (probably not the best way to do this):
Code:
sub EVENT_COMBAT {
if ($combat_state == 1) {
quest::setnexthpevent(75);
}
}
sub EVENT_HP {
if ($hpevent <= 75) {
$x1 = quest::chooserandom(20,-20);
$x2 = $npc->GetX(); - $x1;
$y1 = quest::chooserandom(20,-20);
$y2 = $npc->GetY(); - $y1;
$z1 = quest::chooserandom(20,-20);
$z2 = $npc->GetZ(); - $z1;
quest::spawn(999270,0,0,$x2,$y2,$z2);
}
}
Finally, an NPC assigned to grid 1 in the zone. Note NPC location does not matter here, as grids put it in another xyz location as soon as the NPC spawns.
Code:
sub EVENT_COMBAT {
if ($combat_state == 1) {
quest::setnexthpevent(75);
}
}
sub EVENT_HP {
if ($hpevent <= 75) {
quest::spawn(999270,0,1,0,0,0);
}
}
Please note depending if you use quest::spawn or quest::spawn2, the heading becomes a requirement. Hope this helps.
|
 |
|
 |
 |
|
 |

01-30-2009, 09:43 PM
|
Sarnak
|
|
Join Date: Jul 2005
Location: Ohio
Posts: 72
|
|
So this is basically what I've come up with:
Code:
sub EVENT_COMBAT {
if ($combat_state == 1) {
quest::setnexthpevent(75);
quest::setnexthpevent(50);
quest::setnexthpevent(25);
}
}
sub EVENT_HP {
if ($hpevent <= 75) {
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
elsif ($hpevent <= 50) {
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
elsif ($hpevent <= 25) {
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
}
Now only the 75% event goes off. The 3 adds spawn, but at 50% and 25%, nothing happens. Any solution to this? I was thinking maybe taking out the "elsif"?
__________________
Building Server
Legends of Time - Full Custom/Legit Roleplay, need devs
|
 |
|
 |
 |
|
 |

01-31-2009, 12:23 AM
|
 |
The PEQ Dude
|
|
Join Date: Apr 2003
Location: -
Posts: 1,988
|
|
Try this:
Code:
sub EVENT_COMBAT {
if ($combat_state == 1) {
quest::setnexthpevent(75);
}
}
sub EVENT_HP {
if ($hpevent == 75) {
quest::setnexthpevent(50);
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
elsif ($hpevent == 50) {
quest::setnexthpevent(25);
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
elsif ($hpevent == 25) {
$x1 = $npc->GetX();
$y1 = $npc->GetY();
$z1 = $npc->GetZ();
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
quest::spawn(999270,0,0,$x1,$y1,$z1);
}
}
Last edited by cavedude; 01-31-2009 at 08:26 AM..
|
 |
|
 |

02-06-2009, 12:48 AM
|
Demi-God
|
|
Join Date: May 2007
Posts: 1,032
|
|
want happens if mob drops from 51% to 49% in one shot? will that trigger the $hpevent == 50 part?
also. if mob kills all the players - the hp events needs to be reset back to 75% again
|

02-06-2009, 01:07 AM
|
 |
The PEQ Dude
|
|
Join Date: Apr 2003
Location: -
Posts: 1,988
|
|
Yep, if it skips it will still trigger it.
If the NPC regenerates back to full health it *should* reset everything. However, if it doesn't (or even if it does, best be safe than sorry) I would recommend having the NPC repop x amount of time after it leaves combat. That would certainly reset everything.
|

02-06-2009, 01:38 AM
|
Demi-God
|
|
Join Date: May 2007
Posts: 1,032
|
|
actualy I was thinking that:
-npc agroes - hp event set to 75
-npc deagroes (all players dead) - combat state is 0 again
-players come back and attack again- combat state becomes 1 again AND it SHOULD triger the
if ($combat_state == 1) {
quest::setnexthpevent(75);
}
again and effectivly reset the encounter chain
now, if npc re-trigers abut have not regenerated I belive it will automaticly spawn all the adds betwin 75% and current health.
Let say npc killed all players when it was at 5%.
Players attack npc again when it got to say 20%
Now since combat state has been retrigered it automaticly should pass checks for 75, 50 and 25 hp events and spawn ALL THE ADDS at once.
Is my logic correct?
|
 |
|
 |

02-06-2009, 02:32 AM
|
 |
The PEQ Dude
|
|
Join Date: Apr 2003
Location: -
Posts: 1,988
|
|
The only thing I am unsure of is it may not retroactively go back and spawn everything in the previous events. Think about it, if they come back at 20%, then they wouldn't trigger any more adds, because the script has already gone past all the quest::setnexthpevent. Now, if they came back at 50%, then the 25% spawn should fire off, because the script has to run through that. See what I mean? The script actually has to get to those functions in order to trigger the events, if the NPC is at a HP level lower than the last function, nothing should get triggered. I think the part that is confusing is previously I said it would "skip" properly. That was incorrect in a way. Nothing gets skipped, if the NPC goes from 51% to 49% in one shot, the server will countdown everything inbetween, so 50% will return true. If the NPC STARTS at 50%, then Perl *should* skip down to the 50% event, and not run anything before that, because only 50% has returned true, not 75%.
Of course, this is only my theory. Give it a try, you could be right, I could be right, or we both could be wrong.
|
 |
|
 |

05-22-2009, 06:44 PM
|
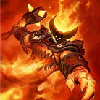 |
Demi-God
|
|
Join Date: Mar 2009
Location: Umm
Posts: 1,492
|
|
I have finaly just tested this- and I was right =)
using the exact code above, if NPC was beaten to say 10% and then disengaged (all opponets killed)
as soon as npc is attacked again (still at 10% hp) he will run ALL the script events which happened at 75, 50 and 25% event all way down
in game this resulted in a whole crap of adds spawned instantly
so once Combat State=1 triger fires- the script effectivly resets and rolls through all events up to current hps
|
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 07:58 AM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |