|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
 |
|
 |

07-11-2010, 09:23 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
COMMITTED: SE_HealRate
I knew there was a cleaner way to do these types of effects but since the SpellVulnerability code was so intertwined with the spell shielding stuff atm, I wanted to do a clean test case on a similar effect. You may remember this effect from fun spells like Balance of Zebuxoruk and paladins will be happy to have their epic 2.0 click working( Flames of the Valiant)
I'm going to go redo the spellvulner code to match this way as I think its much cleaner and of course crits matching dmg is always a bonus. Will post diffs of just the spell shielding code and then the spell effect code as well, to keep it cleaner in that thread. Will have to update the skilldmgtaken effect as well as crits arent matching dmg.
mob.h
Code:
Index: mob.h
===================================================================
--- mob.h (revision 1596)
+++ mob.h (working copy)
@@ -778,6 +778,7 @@
bool TryDeathSave();
void DoBuffWearOffEffect(uint32 index);
void TryTriggerOnCast(Mob *target, uint32 spell_id);
+ int32 GetHealRate(uint32 amount, Mob *target);
static int32 GetAppearanceValue(EmuAppearance iAppearance);
void SendAppearancePacket(int32 type, int32 value, bool WholeZone = true, bool iIgnoreSelf = false, Client *specific_target=NULL);
mob.cpp
Code:
Index: mob.cpp
===================================================================
--- mob.cpp (revision 1596)
+++ mob.cpp (working copy)
@@ -3036,4 +3036,32 @@
}
}
}
+}
+
+int32 Mob::GetHealRate(uint32 amount, Mob *target)
+{
+
+ int slot = target->GetBuffSlotFromType(SE_HealRate);
+ if(slot >= 0)
+ {
+ sint32 modify_amount = amount;
+ for(int i = 0; i < EFFECT_COUNT; i++)
+ {
+ if (spells[buffs[slot].spellid].effectid[i] == SE_HealRate)
+ {
+ // if the effect reduces the heal amount below 0, return 0.
+ if(spells[buffs[slot].spellid].base[i] < -100)
+ {
+ amount = 0;
+ break;
+ }
+ else
+ {
+ amount += (modify_amount * spells[buffs[slot].spellid].base[i] / 100);
+ break;
+ }
+ }
+ }
+ }
+ return amount;
}
\ No newline at end of file
spell effects.cpp
Code:
Index: spell_effects.cpp
===================================================================
--- spell_effects.cpp (revision 1596)
+++ spell_effects.cpp (working copy)
@@ -211,8 +211,10 @@
else if(dmg > 0) {
//healing spell...
if(caster)
+ {
+ dmg = GetHealRate(dmg, caster->GetTarget());
dmg = caster->GetActSpellHealing(spell_id, dmg);
-
+ }
HealDamage(dmg, caster);
}
@@ -248,6 +250,8 @@
dmg = -dmg;
Damage(caster, dmg, spell_id, spell.skill, false, buffslot, false);
} else {
+ if(caster)
+ dmg = GetHealRate(dmg, caster->GetTarget());
HealDamage(dmg, caster);
}
break;
@@ -272,6 +276,8 @@
val = cap;
if(val > 0)
+ if(caster)
+ val = GetHealRate(val, caster->GetTarget());
HealDamage(val, caster);
break;
@@ -2209,17 +2215,6 @@
break;
}
- case SE_HealRate:
- {
-#ifdef SPELL_EFFECT_SPAM
- snprintf(effect_desc, _EDLEN, "Heal Effectiveness: %d%%", effect_value);
-#endif
- // solar: TODO implement this
- const char *msg = "Heal Effectiveness is not implemented.";
- if(caster) caster->Message(13, msg);
- break;
- }
-
case SE_Screech:
{
#ifdef SPELL_EFFECT_SPAM
@@ -2812,6 +2807,7 @@
case SE_LimitCastTime:
case SE_NoCombatSkills:
case SE_TriggerOnCast:
+ case SE_HealRate:
{
break;
}
@@ -3143,7 +3139,10 @@
//healing spell...
//healing aggro would go here; removed for now
if(caster)
+ {
+ effect_value = GetHealRate(effect_value, caster->GetTarget());
effect_value = caster->GetActSpellHealing(spell_id, effect_value);
+ }
HealDamage(effect_value, caster);
}
@@ -3151,8 +3150,9 @@
}
case SE_HealOverTime:
{
+
effect_value = CalcSpellEffectValue(spell_id, i, caster_level);
-
+ effect_value = GetHealRate(effect_value, this);
//is this affected by stuff like GetActSpellHealing??
HealDamage(effect_value, caster);
//healing aggro would go here; removed for now
|
 |
|
 |

07-13-2010, 12:17 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Couple suggestions:
spell effects.cpp
Code:
@@ -272,6 +276,8 @@
val = cap;
if(val > 0)
+ if(caster)
+ val = GetHealRate(val, caster->GetTarget());
HealDamage(val, caster);
break;
I assume you intended this instead:
Code:
if(val > 0)
{
if(caster && caster->GetTarget())
val = GetHealRate(val, caster->GetTarget());
HealDamage(val, caster);
}
Needed the brackets surrounding it and I added a check to make sure the caster has a target before sending them to the function that does not appear to check for a null pointer in the target. Those null pointers can be nasty :P
|

07-15-2010, 08:34 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
I am still testing most of the effects you have submitted. I haven't been able to test this one or the skill attack one yet due to some crash issues in the latest source updates. I wanted to mention here that I was also getting some crashes that seemed to be caused by the heal code here. I think they were just related to a potential null pointer that is easily fixed by doing something like this:
Code:
@@ -3143,7 +3139,10 @@
//healing spell...
//healing aggro would go here; removed for now
if(caster)
+ {
+ if(caster->GetTarget())
+ effect_value = GetHealRate(effect_value, caster->GetTarget());
effect_value = caster->GetActSpellHealing(spell_id, effect_value);
+ }
HealDamage(effect_value, caster);
}
|
 |
|
 |

07-15-2010, 10:17 AM
|
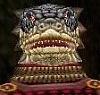 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Quote:
Originally Posted by trevius
I am still testing most of the effects you have submitted. I haven't been able to test this one or the skill attack one yet due to some crash issues in the latest source updates. I wanted to mention here that I was also getting some crashes that seemed to be caused by the heal code here. I think they were just related to a potential null pointer that is easily fixed by doing something like this:
Code:
@@ -3143,7 +3139,10 @@
//healing spell...
//healing aggro would go here; removed for now
if(caster)
+ {
+ if(caster->GetTarget())
+ effect_value = GetHealRate(effect_value, caster->GetTarget());
effect_value = caster->GetActSpellHealing(spell_id, effect_value);
+ }
HealDamage(effect_value, caster);
}
|
Yeah, unfortunately there's no way to get a caster out of zone, so this is the best solution for now; same issues I ran into with Mythic, Timesfive, etc, when doing the spelldamage/healing code. It may be worth mentioning that it's probably better to add GetHealRate into GetActSpellHealing instead of that function. Also, it may be worth looking into making an NPC::GetActSpellHealing, for those times when NPCs calculate differently.
|
 |
|
 |

07-16-2010, 09:36 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
I think it was that NPCs don't change targets when self buffing, as far as I know. So, when NPCs started self buffing on zone startup and cast certain buffs that might run through this code, it crashed the zone. At least that is my best guess. It was definitely NPCs causing it.
Finally got to test it again with the latest source and it no longer crashes with the few changes I have made.
|

07-16-2010, 08:01 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
thanks for the testing Trev, hopefully we can get these effects solid pretty soon.
|
 |
|
 |

11-05-2010, 02:19 AM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
Rewrote this effect as well to use the bonus system as well as being incorporated into GetActSpellHealing.
Code:
Index: EQEmuServer/zone/bonuses.cpp
===================================================================
--- EQEmuServer/zone/bonuses.cpp (revision 1713)
+++ EQEmuServer/zone/bonuses.cpp (working copy)
@@ -1206,8 +1206,11 @@
newbon->Accuracy = effect_value;
break;
}
+ case SE_HealRate:
+ {
+ newbon->HealRate += effect_value;
+ break;
+ }
}
}
}
Index: EQEmuServer/zone/effects.cpp
===================================================================
--- EQEmuServer/zone/effects.cpp (revision 1713)
+++ EQEmuServer/zone/effects.cpp (working copy)
@@ -219,12 +219,22 @@
}
sint32 Client::GetActSpellHealing(int16 spell_id, sint32 value) {
+
sint32 modifier = 100;
modifier += GetFocusEffect(focusImprovedHeal, spell_id);
-
+
+ // Instant Heals
if(spells[spell_id].buffduration < 1) {
- //non-dot
+
+ // Check for buffs that affect the healrate of the target
+ if (this->GetTarget())
+ {
+ value += value * GetHealRate(this->GetTarget()) / 100;
+ if(value < 1)
+ return 0;
+ }
+
switch(GetAA(aaHealingAdept)) {
case 1:
modifier += 2;
Index: EQEmuServer/zone/mob.cpp
===================================================================
--- EQEmuServer/zone/mob.cpp (revision 1713)
+++ EQEmuServer/zone/mob.cpp (working copy)
@@ -3323,34 +3323,12 @@
return damage;
}
-int32 Mob::GetHealRate(uint32 amount, Mob *target)
+int32 Mob::GetHealRate(Mob *target)
{
-
- if(target) {
- int slot = target->GetBuffSlotFromType(SE_HealRate);
- if(slot >= 0)
- {
- sint32 modify_amount = amount;
- for(int i = 0; i < EFFECT_COUNT; i++)
- {
- if (spells[buffs[slot].spellid].effectid[i] == SE_HealRate)
- {
- // if the effect reduces the heal amount below 0, return 0.
- if(spells[buffs[slot].spellid].base[i] < -100)
- {
- amount = 0;
- break;
- }
- else
- {
- amount += (modify_amount * spells[buffs[slot].spellid].base[i] / 100);
- break;
- }
- }
- }
- }
- }
- return amount;
+ if(!target)
+ return 0;
+
+ return (target->itembonuses.HealRate + target->spellbonuses.HealRate);
}
bool Mob::TryFadeEffect(int slot)
Index: EQEmuServer/zone/mob.h
===================================================================
--- EQEmuServer/zone/mob.h (revision 1713)
+++ EQEmuServer/zone/mob.h (working copy)
@@ -275,6 +275,7 @@
sint8 HundredHands; //extra haste, stacks with all other haste i
sint8 MeleeLifetap;
+ sint16 HealRate;
int XPRateMod;
sint8 Packrat; //weight reduction for items, 1 point = 10%
@@ -796,7 +797,7 @@
void TryTwincast(Mob *caster, Mob *target, uint32 spell_id);
void TrySympatheticProc(Mob *target, uint32 spell_id);
bool TryFadeEffect(int slot);
- int32 GetHealRate(uint32 amount, Mob *target);
+ int32 GetHealRate(Mob *target);
sint32 GetVulnerability(sint32 damage, Mob *caster, uint32 spell_id, int32 ticsremaining);
sint32 GetSkillDmgTaken(const SkillType skill_used, sint32 damage);
void DoKnockback(Mob *caster, uint32 pushback, uint32 pushup);
Index: EQEmuServer/zone/spell_effects.cpp
===================================================================
--- EQEmuServer/zone/spell_effects.cpp (revision 1713)
+++ EQEmuServer/zone/spell_effects.cpp (working copy)
@@ -213,10 +213,8 @@
else if(dmg > 0) {
//healing spell...
if(caster)
- {
- dmg = GetHealRate(dmg, caster->GetTarget());
dmg = caster->GetActSpellHealing(spell_id, dmg);
- }
+
HealDamage(dmg, caster);
}
@@ -252,8 +250,6 @@
dmg = -dmg;
Damage(caster, dmg, spell_id, spell.skill, false, buffslot, false);
} else {
- if(caster)
- dmg = GetHealRate(dmg, caster->GetTarget());
HealDamage(dmg, caster);
}
break;
@@ -278,11 +274,7 @@
val = cap;
if(val > 0)
- {
- if(caster && caster->GetTarget())
- val = GetHealRate(val, caster->GetTarget());
HealDamage(val, caster);
- }
break;
}
@@ -3246,25 +3238,20 @@
effect_value = -effect_value;
Damage(caster, effect_value, spell_id, spell.skill, false, i, true);
} else if(effect_value > 0) {
- //healing spell...
- //healing aggro would go here; removed for now
- if(caster)
- {
- if (caster->GetTarget())
- effect_value = GetHealRate(effect_value, caster->GetTarget());
- effect_value = caster->GetActSpellHealing(spell_id, effect_value);
- }
+ // Regen spell...
+ effect_value += effect_value * (itembonuses.HealRate + spellbonuses.HealRate) / 100;
HealDamage(effect_value, caster);
}
-
break;
}
case SE_HealOverTime:
{
effect_value = CalcSpellEffectValue(spell_id, i, caster_level);
- effect_value = GetHealRate(effect_value, this);
- //is this affected by stuff like GetActSpellHealing??
+ if(caster)
+ // Currently returns no change as there are no AAs that affect HoTs but soon.
+ effect_value = caster->GetActSpellHealing(spell_id, effect_value);
+ effect_value += effect_value * (itembonuses.HealRate + spellbonuses.HealRate) / 100;
HealDamage(effect_value, caster);
//healing aggro would go here; removed for now
break;
|
 |
|
 |
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 05:50 AM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |