|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
 |
|
 |

10-04-2010, 01:50 PM
|
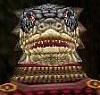 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
PARTIALLY COMMITTED: New Perl Exported Functions
This will add SetMinDamage, SetMaxDamage, SetAccuracyRating, SetBaseHP, and GetMinDMG to perl for NPCs, and GetIP to perl for clients.
SetMinDamage, etc, doesn't change it on the database, only for that specific NPC. The point in exporting these is so you could in theory scale entire zones to level when npcs spawn.
You can do this by getting a client list whenever someone zones in and scale the NPCs appropriately. Akkadius had asked me to implement this so I did.
I left basehp setting in NPC, because well, do we really need to set the base hp of clients, especially since they don't show properly?
Code:
Index: zone/npc.cpp
===================================================================
--- zone/npc.cpp (revision 1688)
+++ zone/npc.cpp (working copy)
@@ -1890,7 +1890,32 @@
return;
}
+void NPC::SetMinDamage(int32 val) {
+ if(val)
+ min_dmg = val;
+ else
+ min_dmg = min_dmg;
+}
+void NPC::SetMaxDamage(int32 val) {
+ if(val)
+ max_dmg = val;
+ else
+ max_dmg = max_dmg;
+}
+void NPC::SetAccuracyRating(int32 val) {
+ if(val)
+ accuracy_rating = accuracy_rating;
+ else
+ accuracy_rating = accuracy_rating;
+}
+void NPC::SetBaseHP(int32 val) { // this should really be Mob::SetBaseHP, but since it wouldn't display on the client, let's put it here
+ if(val)
+ base_hp = val;
+ else
+ base_hp = base_hp;
+}
+
int32 NPC::GetSpawnPointID() const
{
if(respawn2)
Index: zone/npc.h
===================================================================
--- zone/npc.h (revision 1688)
+++ zone/npc.h (working copy)
@@ -213,7 +213,8 @@
float org_x, org_y, org_z, org_heading;
- int16 GetMaxDMG() const {return max_dmg;}
+ int32 GetMinDMG() const {return min_dmg;}
+ int32 GetMaxDMG() const {return max_dmg;}
bool IsAnimal() const { return(bodytype == BT_Animal); }
int16 GetPetSpellID() const {return pet_spell_id;}
void SetPetSpellID(int16 amt) {pet_spell_id = amt;}
@@ -306,6 +307,11 @@
uint32 GetAdventureTemplate() const { return adventure_template_id; }
+ void SetMinDamage(int32 val);
+ void SetMaxDamage(int32 val);
+ void SetAccuracyRating(int32 val);
+ void SetBaseHP(int32 val);
+
protected:
const NPCType* NPCTypedata;
Index: zone/perl_client.cpp
===================================================================
--- zone/perl_client.cpp (revision 1688)
+++ zone/perl_client.cpp (working copy)
@@ -4590,6 +4590,33 @@
}
+XS(XS_Client_GetIP); /* prototype to pass -Wmissing-prototypes */
+XS(XS_Client_GetIP)
+{
+ dXSARGS;
+ if (items != 1)
+ Perl_croak(aTHX_ "Usage: Client::GetIP(THIS)");
+ {
+ Client * THIS;
+ int32 RETVAL;
+ dXSTARG;
+
+ if (sv_derived_from(ST(0), "Client")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(Client *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type Client");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ RETVAL = THIS->GetIP();
+ XSprePUSH; PUSHu((UV)RETVAL);
+ }
+ XSRETURN(1);
+}
+
+
#ifdef __cplusplus
extern "C"
#endif
@@ -4779,6 +4806,7 @@
newXSproto(strcpy(buf, "SetEndurance"), XS_Client_SetEndurance, file, "$$");
newXSproto(strcpy(buf, "SendOPTranslocateConfirm"), XS_Client_SendOPTranslocateConfirm, file, "$$$");
newXSproto(strcpy(buf, "NPCSpawn"), XS_Client_NPCSpawn, file, "$$$;$");
+ newXSproto(strcpy(buf, "GetIP"), XS_Client_GetIP, file, "$");
XSRETURN_YES;
}
Index: zone/perl_npc.cpp
===================================================================
--- zone/perl_npc.cpp (revision 1688)
+++ zone/perl_npc.cpp (working copy)
@@ -814,7 +814,7 @@
Perl_croak(aTHX_ "Usage: NPC::GetMaxDMG(THIS)");
{
NPC * THIS;
- int16 RETVAL;
+ int32 RETVAL;
dXSTARG;
if (sv_derived_from(ST(0), "NPC")) {
@@ -1866,6 +1866,131 @@
XSRETURN_EMPTY;
}
+XS(XS_NPC_SetMinDamage); /* prototype to pass -Wmissing-prototypes */
+XS(XS_NPC_SetMinDamage)
+{
+ dXSARGS;
+ if (items != 2)
+ Perl_croak(aTHX_ "Usage: NPC::SetMinDamage(THIS, amt)");
+ {
+ NPC * THIS;
+ int32 amt = (int16)SvUV(ST(1));
+
+ if (sv_derived_from(ST(0), "NPC")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(NPC *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type NPC");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ THIS->SetMinDamage(amt);
+ }
+ XSRETURN_EMPTY;
+}
+
+XS(XS_NPC_SetMaxDamage); /* prototype to pass -Wmissing-prototypes */
+XS(XS_NPC_SetMaxDamage)
+{
+ dXSARGS;
+ if (items != 2)
+ Perl_croak(aTHX_ "Usage: NPC::SetMaxDamage(THIS, amt)");
+ {
+ NPC * THIS;
+ int32 amt = (int16)SvUV(ST(1));
+
+ if (sv_derived_from(ST(0), "NPC")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(NPC *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type NPC");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ THIS->SetMaxDamage(amt);
+ }
+ XSRETURN_EMPTY;
+}
+
+XS(XS_NPC_SetAccuracyRating); /* prototype to pass -Wmissing-prototypes */
+XS(XS_NPC_SetAccuracyRating)
+{
+ dXSARGS;
+ if (items != 2)
+ Perl_croak(aTHX_ "Usage: NPC::SetAccuracyRating(THIS, amt)");
+ {
+ NPC * THIS;
+ int32 amt = (int16)SvUV(ST(1));
+
+ if (sv_derived_from(ST(0), "NPC")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(NPC *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type NPC");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ THIS->SetAccuracyRating(amt);
+ }
+ XSRETURN_EMPTY;
+}
+
+XS(XS_NPC_SetBaseHP); /* prototype to pass -Wmissing-prototypes */
+XS(XS_NPC_SetBaseHP)
+{
+ dXSARGS;
+ if (items != 2)
+ Perl_croak(aTHX_ "Usage: NPC::SetBaseHP(THIS, amt)");
+ {
+ NPC * THIS;
+ int32 amt = (int16)SvUV(ST(1));
+
+ if (sv_derived_from(ST(0), "NPC")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(NPC *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type NPC");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ THIS->SetBaseHP(amt);
+ }
+ XSRETURN_EMPTY;
+}
+
+
+XS(XS_NPC_GetMinDMG); /* prototype to pass -Wmissing-prototypes */
+XS(XS_NPC_GetMinDMG)
+{
+ dXSARGS;
+ if (items != 1)
+ Perl_croak(aTHX_ "Usage: NPC::GetMinDMG(THIS)");
+ {
+ NPC * THIS;
+ int32 RETVAL;
+ dXSTARG;
+
+ if (sv_derived_from(ST(0), "NPC")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(NPC *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type NPC");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ RETVAL = THIS->GetMinDMG();
+ XSprePUSH; PUSHu((UV)RETVAL);
+ }
+ XSRETURN(1);
+}
+
+
+
#ifdef __cplusplus
extern "C"
#endif
@@ -1956,6 +2081,11 @@
newXSproto(strcpy(buf, "GetSwarmTarget"), XS_NPC_GetSwarmTarget, file, "$");
newXSproto(strcpy(buf, "SetSwarmTarget"), XS_NPC_SetSwarmTarget, file, "$$");
newXSproto(strcpy(buf, "ModifyNPCStat"), XS_NPC_ModifyNPCStat, file, "$$$");
+ newXSproto(strcpy(buf, "SetMinDamage"), XS_NPC_SetMinDamage, file, "$$");
+ newXSproto(strcpy(buf, "SetMaxDamage"), XS_NPC_SetMaxDamage, file, "$$");
+ newXSproto(strcpy(buf, "SetAccuracyRating"), XS_NPC_SetAccuracyRating, file, "$$");
+ newXSproto(strcpy(buf, "SetBaseHP"), XS_NPC_SetBaseHP, file, "$$");
+ newXSproto(strcpy(buf, "GetMinDMG"), XS_NPC_GetMinDMG, file, "$");
XSRETURN_YES;
}
|
 |
|
 |
 |
|
 |

10-04-2010, 03:09 PM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Quote:
Originally Posted by Secrets
This will add SetMinDamage, SetMaxDamage, SetAccuracyRating, SetBaseHP, and GetMinDMG to perl for NPCs, and GetIP to perl for clients.
|
Most of those commands are already covered by either quest::modifynpcstat(identifier, value) or the NPC object ModifyNPCStat(identifier, newValue).
Here are the list of identifiers that can be modified:
http://www.eqemulator.net/wiki/wikka...tofIdentifiers
I am not sure what SetBaseHP does that SetMaxHP() and/or SetHP() can't do.
I think that leaves the GetMinDMG and GetIP as valid submissions that we can use. I think GetIP is definitely a good one to have at least. Thanks for that.
If we want to pull NPC stats that don't already have functions for them, we could probably make a command that works similar to the ModifyNPCStat() command that you just enter an identifier of what you want, so we can save from having 30 new functions.
Maybe I am missing something here. Not trying to criticize your submission, I just want to make sure we aren't duplicating stuff that already exists.
|
 |
|
 |
 |
|
 |

10-04-2010, 03:31 PM
|
 |
Administrator
|
|
Join Date: Feb 2009
Location: MN
Posts: 2,071
|
|
Quote:
Originally Posted by trevius
Most of those commands are already covered by either quest::modifynpcstat(identifier, value) or the NPC object ModifyNPCStat(identifier, newValue).
Here are the list of identifiers that can be modified:
http://www.eqemulator.net/wiki/wikka...tofIdentifiers
I am not sure what SetBaseHP does that SetMaxHP() and/or SetHP() can't do.
I think that leaves the GetMinDMG and GetIP as valid submissions that we can use. I think GetIP is definitely a good one to have at least. Thanks for that.
If we want to pull NPC stats that don't already have functions for them, we could probably make a command that works similar to the ModifyNPCStat() command that you just enter an identifier of what you want, so we can save from having 30 new functions.
Maybe I am missing something here. Not trying to criticize your submission, I just want to make sure we aren't duplicating stuff that already exists.
|
I kind of was tossing some light chat with Secrets and didn't think she'd post this right away. But I thought IP was a good thing to have considering you can limit rewards based on toons in the same zone with the same IP.
|
 |
|
 |

10-04-2010, 03:32 PM
|
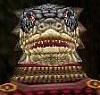 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Currently SetMaxHP calculates the max hp and nothing else. It does not set the BASE hp (the thing loaded from the DB) to a proper value. I see that's already a 'max_value' in ModifyNPStat, so that can be discarded.
As for damage I had no idea, thanks for showing me that.
|

10-04-2010, 03:34 PM
|
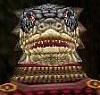 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Yeah I just saw the NPC::ModifyNPCStat function. Oops. Feel free to take getmindamage and getIP though.
|

10-05-2010, 07:51 AM
|
Developer
|
|
Join Date: Mar 2009
Location: -
Posts: 228
|
|
Those two are worth their weight in gold either way. Thanks!
|
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 11:59 PM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |