|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
 |
|
 |

10-14-2012, 05:42 PM
|
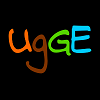 |
Developer
|
|
Join Date: Apr 2012
Location: North Carolina
Posts: 2,815
|
|
#peekinv money
A quick little patch for anyone interested in viewing a player's money.
It uses currently coded public client functions, but could be expounded upon if the need ever arises.
[MoneyPeek.patch]
Code:
Index: command.cpp
===================================================================
--- command.cpp (revision 2229)
+++ command.cpp (working copy)
@@ -263,7 +263,7 @@
command_add("appearance","[type] [value] - Send an appearance packet for you or your target",150,command_appearance) ||
command_add("charbackup","[list/restore] - Query or restore character backups",150,command_charbackup) ||
command_add("nukeitem","[itemid] - Remove itemid from your player target's inventory",150,command_nukeitem) ||
- command_add("peekinv","[worn/cursor/inv/bank/trade/trib/all] - Print out contents of your player target's inventory",100,command_peekinv) ||
+ command_add("peekinv","[money/worn/cursor/inv/bank/trade/trib/all] - Print out contents of your player target's inventory",100,command_peekinv) ||
command_add("findnpctype","[search criteria] - Search database NPC types",100,command_findnpctype) ||
command_add("findzone","[search criteria] - Search database zones",100,command_findzone) ||
command_add("fz",NULL,100,command_findzone) ||
@@ -3029,7 +3029,18 @@
Client* client = c->GetTarget()->CastToClient();
const Item_Struct* item = NULL;
c->Message(0, "Displaying inventory for %s...", client->GetName());
-
+
+ if (bAll || (strcasecmp(sep->arg[1], "money")==0)) {
+ // Money
+ bFound = true;
+ c->Message(0, "Total Money (in Copper): %i", client->GetAllMoney());
+ c->Message(0, "Carried Money (in Copper): %i", client->GetCarriedMoney());
+ c->Message(0, "Carried Platinum: %i", client->GetPlatinum());
+ c->Message(0, "Carried Gold: %i", client->GetGold());
+ c->Message(0, "Carried Silver: %i", client->GetSilver());
+ c->Message(0, "Carried Copper: %i", client->GetCopper());
+ }
+
if (bAll || (strcasecmp(sep->arg[1], "worn")==0)) {
// Worn items
bFound = true;
@@ -3331,7 +3342,7 @@
if (!bFound)
{
- c->Message(0, "Usage: #peekinv [worn|cursor|inv|bank|trade|trib|all]");
+ c->Message(0, "Usage: #peekinv [money|worn|cursor|inv|bank|trade|trib|all]");
c->Message(0, " Displays a portion of the targeted user's inventory");
c->Message(0, " Caution: 'all' is a lot of information!");
}
__________________
Uleat of Bertoxxulous
Compilin' Dirty
|
 |
|
 |
 |
|
 |

10-16-2012, 09:19 PM
|
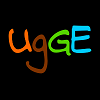 |
Developer
|
|
Join Date: Apr 2012
Location: North Carolina
Posts: 2,815
|
|
Sorry guys! I hadn't planned on adding this stuff myself, but I hit a manic state the other night and this is the outcome...
I added Lerxst's inferred change for the AddMoneyToPP(), which is pretty sweet! I also added the needed code required to bring the '#peekinv money' command
into full maturation.
Additionally, I totally reworked TakeMoneyFromPP() to eliminate most of the overhead created by the previous incarnation. (I broke my cardinal rule and my
apologies if I offend anyone with this change.)
This tested ok on my system, but if anyone would like a less radical version (sans TMFPP()) of this patch, just let me know.
THIS IS AN ALPHA PATCH!
Please review and test it on a private/test server before going live.
(I don't know why my revision is showing 2229..the file is rev 2209 and the source is the current 2226.)
[MoneyUpdate.patch]
Code:
Index: client.cpp
===================================================================
--- client.cpp (revision 2229)
+++ client.cpp (working copy)
@@ -2024,182 +2024,109 @@
}
bool Client::TakeMoneyFromPP(uint64 copper, bool updateclient) {
- sint64 copperpp,silver,gold,platinum;
- copperpp = m_pp.copper;
- silver = static_cast<sint64>(m_pp.silver) * 10;
- gold = static_cast<sint64>(m_pp.gold) * 100;
- platinum = static_cast<sint64>(m_pp.platinum) * 1000;
+ // This procedure was previously mathematically intense. This should return a more contant rate result
+ // without the additional math checks as denominational inclusion increases -U
- sint64 clienttotal = copperpp + silver + gold + platinum;
+ if (!HasMoney(copper)) { return false; } // code already exists..let's use it
- clienttotal -= copper;
- if(clienttotal < 0)
- {
- return false; // Not enough money!
+ sint64 cur_debt = copper;
+ sint64 cur_copper = m_pp.copper;
+ sint64 cur_silver = m_pp.silver;
+ sint64 cur_gold = m_pp.gold;
+ sint64 cur_platinum = m_pp.platinum;
+
+ // Subtract from Copper
+ cur_copper -= cur_debt; // subtract debt from player copper
+ if (cur_copper < 0) { // if debt exceeds copper amount...
+ cur_debt = abs64(cur_copper); // reassign the difference of debt and copper
+ if ((cur_debt % CONVERSION_RATE) > 0) { // if there's a left-over of copper debt...
+ cur_copper = (CONVERSION_RATE - (cur_debt % CONVERSION_RATE)); // borrow to clear left-over
+ cur_debt += cur_copper; // add borrowed back to debt
+ }
}
- else
- {
- copperpp -= copper;
- if(copperpp <= 0)
- {
- copper = abs64(copperpp);
- m_pp.copper = 0;
+ else { cur_debt = 0; } // if player copper payed everything, then clear debt
+
+ // Subtract from Silver
+ cur_debt /= CONVERSION_RATE; // convert copper to silver (copper place value was zeroed previously)
+ cur_silver -= cur_debt; // rinse, repeat
+ if (cur_silver < 0) {
+ cur_debt = abs64(cur_silver);
+ if ((cur_debt % CONVERSION_RATE) > 0) {
+ cur_silver = (CONVERSION_RATE - (cur_debt % CONVERSION_RATE));
+ cur_debt += cur_silver;
}
- else
- {
- m_pp.copper = copperpp;
- if(updateclient)
- SendMoneyUpdate();
- Save();
- return true;
+ }
+ else { cur_debt = 0; }
+
+ // Subtract from Gold
+ cur_debt /= CONVERSION_RATE;
+ cur_gold -= cur_debt;
+ if (cur_gold < 0) {
+ cur_debt = abs64(cur_gold);
+ if ((cur_debt % CONVERSION_RATE) > 0) {
+ cur_gold = (CONVERSION_RATE - (cur_debt % CONVERSION_RATE));
+ cur_debt += cur_gold;
}
- silver -= copper;
- if(silver <= 0)
- {
- copper = abs64(silver);
- m_pp.silver = 0;
- }
- else
- {
- m_pp.silver = silver/10;
- m_pp.copper += (silver-(m_pp.silver*10));
- if(updateclient)
- SendMoneyUpdate();
- Save();
- return true;
- }
+ }
+ else { cur_debt = 0; }
+
+ // Subtract from Platinum
+ cur_debt /= CONVERSION_RATE;
+ cur_platinum -= cur_debt;
+ if (cur_platinum < 0) { // just in case something went wrong, let's log it if debug level is set and zero the fault
+
+#if (EQDEBUG>=5)
+ LogFile->write(EQEMuLog::Debug, "Client::TakeMoneyFromPP() %s's transaction resulted in a deficit of %i platinum",
+ GetName(), cur_platinum);
+#endif
- gold -=copper;
+ cur_platinum = 0;
+ }
- if(gold <= 0)
- {
- copper = abs64(gold);
- m_pp.gold = 0;
- }
- else
- {
- m_pp.gold = gold/100;
- int64 silvertest = (gold-(static_cast<int64>(m_pp.gold)*100))/10;
- m_pp.silver += silvertest;
- int64 coppertest = (gold-(static_cast<int64>(m_pp.gold)*100+silvertest*10));
- m_pp.copper += coppertest;
- if(updateclient)
- SendMoneyUpdate();
- Save();
- return true;
- }
-
- platinum -= copper;
-
- //Impossible for plat to be negative, already checked above
-
- m_pp.platinum = platinum/1000;
- int64 goldtest = (platinum-(static_cast<int64>(m_pp.platinum)*1000))/100;
- m_pp.gold += goldtest;
- int64 silvertest = (platinum-(static_cast<int64>(m_pp.platinum)*1000+goldtest*100))/10;
- m_pp.silver += silvertest;
- int64 coppertest = (platinum-(static_cast<int64>(m_pp.platinum)*1000+goldtest*100+silvertest*10));
- m_pp.copper = coppertest;
- if(updateclient)
- SendMoneyUpdate();
- RecalcWeight();
- Save();
- return true;
- }
+ m_pp.copper = cur_copper;
+ m_pp.silver = cur_silver;
+ m_pp.gold = cur_gold;
+ m_pp.platinum = cur_platinum;
+
+ if (updateclient) { SendMoneyUpdate(); }
+
+ RecalcWeight();
+ Save();
+ return true;
}
void Client::AddMoneyToPP(uint64 copper, bool updateclient){
- uint64 tmp;
- uint64 tmp2;
- tmp = copper;
+ // I added the code that lerxst provided - this is pretty tight! -U
- // Add Amount of Platinum
- tmp2 = tmp/1000;
- sint32 new_val = m_pp.platinum + tmp2;
- if(new_val < 0) {
- m_pp.platinum = 0;
- } else {
- m_pp.platinum = m_pp.platinum + tmp2;
- }
- tmp-=tmp2*1000;
-
- //if (updateclient)
- // SendClientMoneyUpdate(3,tmp2);
-
- // Add Amount of Gold
- tmp2 = tmp/100;
- new_val = m_pp.gold + tmp2;
- if(new_val < 0) {
- m_pp.gold = 0;
- } else {
- m_pp.gold = m_pp.gold + tmp2;
- }
- tmp-=tmp2*100;
- //if (updateclient)
- // SendClientMoneyUpdate(2,tmp2);
-
- // Add Amount of Silver
- tmp2 = tmp/10;
- new_val = m_pp.silver + tmp2;
- if(new_val < 0) {
- m_pp.silver = 0;
- } else {
- m_pp.silver = m_pp.silver + tmp2;
- }
- tmp-=tmp2*10;
- //if (updateclient)
- // SendClientMoneyUpdate(1,tmp2);
-
- // Add Copper
- //tmp = tmp - (tmp2* 10);
- //if (updateclient)
- // SendClientMoneyUpdate(0,tmp);
- new_val = m_pp.copper + tmp2;
- if(new_val < 0) {
- m_pp.copper = 0;
- } else {
- m_pp.copper = m_pp.copper + tmp2;
- }
-
-
- //send them all at once, since the above code stopped working.
- if(updateclient)
- SendMoneyUpdate();
-
- RecalcWeight();
-
- Save();
-
- LogFile->write(EQEMuLog::Debug, "Client::AddMoneyToPP() %s should have: plat:%i gold:%i silver:%i copper:%i", GetName(), m_pp.platinum, m_pp.gold, m_pp.silver, m_pp.copper);
+ sint32 platinum = (copper / PLATINUM_RATE);
+ sint32 gold = (copper / GOLD_RATE) % 10;
+ sint32 silver = (copper / SILVER_RATE) % 10;
+ copper %= 10;
+ AddMoneyToPP(copper, silver, gold, platinum, updateclient);
}
void Client::AddMoneyToPP(uint32 copper, uint32 silver, uint32 gold, uint32 platinum, bool updateclient){
-
- sint32 new_value = m_pp.platinum + platinum;
- if(new_value >= 0 && new_value > m_pp.platinum)
- m_pp.platinum += platinum;
- new_value = m_pp.gold + gold;
- if(new_value >= 0 && new_value > m_pp.gold)
- m_pp.gold += gold;
+ sint32 new_value = m_pp.platinum + platinum;
+ if(new_value >= 0 && new_value > m_pp.platinum) { m_pp.platinum += platinum; }
- new_value = m_pp.silver + silver;
- if(new_value >= 0 && new_value > m_pp.silver)
- m_pp.silver += silver;
+ new_value = m_pp.gold + gold;
+ if(new_value >= 0 && new_value > m_pp.gold) { m_pp.gold += gold; }
- new_value = m_pp.copper + copper;
- if(new_value >= 0 && new_value > m_pp.copper)
- m_pp.copper += copper;
+ new_value = m_pp.silver + silver;
+ if(new_value >= 0 && new_value > m_pp.silver) { m_pp.silver += silver; }
- if(updateclient)
- SendMoneyUpdate();
+ new_value = m_pp.copper + copper;
+ if(new_value >= 0 && new_value > m_pp.copper) { m_pp.copper += copper; }
+ if(updateclient) { SendMoneyUpdate(); }
+
RecalcWeight();
Save();
#if (EQDEBUG>=5)
- LogFile->write(EQEMuLog::Debug, "Client::AddMoneyToPP() %s should have: plat:%i gold:%i silver:%i copper:%i",
- GetName(), m_pp.platinum, m_pp.gold, m_pp.silver, m_pp.copper);
+ LogFile->write(EQEMuLog::Debug, "Client::AddMoneyToPP() %s should have: plat:%i gold:%i silver:%i copper:%i",
+ GetName(), m_pp.platinum, m_pp.gold, m_pp.silver, m_pp.copper);
#endif
}
@@ -2218,9 +2145,9 @@
bool Client::HasMoney(uint64 Copper) {
if((static_cast<int64>(m_pp.copper) +
- (static_cast<int64>(m_pp.silver) * 10) +
- (static_cast<int64>(m_pp.gold) * 100) +
- (static_cast<int64>(m_pp.platinum) * 1000)) >= Copper)
+ (static_cast<int64>(m_pp.silver) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum) * PLATINUM_RATE)) >= Copper)
return true;
return false;
@@ -2228,28 +2155,53 @@
int64 Client::GetCarriedMoney() {
- return ((static_cast<int64>(m_pp.copper) +
- (static_cast<int64>(m_pp.silver) * 10) +
- (static_cast<int64>(m_pp.gold) * 100) +
- (static_cast<int64>(m_pp.platinum) * 1000)));
+ return (
+ (static_cast<int64>(m_pp.copper)) +
+ (static_cast<int64>(m_pp.silver) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum) * PLATINUM_RATE));
}
+int64 Client::GetBankMoney() {
+
+ return (
+ (static_cast<int64>(m_pp.copper_bank)) +
+ (static_cast<int64>(m_pp.silver_bank) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold_bank) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum_bank) * PLATINUM_RATE));
+}
+
+int64 Client::GetSharedBankMoney() {
+
+ return (
+ (static_cast<int64>(m_pp.platinum_shared) * PLATINUM_RATE));
+}
+
+int64 Client::GetCursorMoney() {
+
+ return (
+ (static_cast<int64>(m_pp.copper_cursor)) +
+ (static_cast<int64>(m_pp.silver_cursor) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold_cursor) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum_cursor) * PLATINUM_RATE));
+}
+
int64 Client::GetAllMoney() {
return (
- (static_cast<int64>(m_pp.copper) +
- (static_cast<int64>(m_pp.silver) * 10) +
- (static_cast<int64>(m_pp.gold) * 100) +
- (static_cast<int64>(m_pp.platinum) * 1000) +
- (static_cast<int64>(m_pp.copper_bank) +
- (static_cast<int64>(m_pp.silver_bank) * 10) +
- (static_cast<int64>(m_pp.gold_bank) * 100) +
- (static_cast<int64>(m_pp.platinum_bank) * 1000) +
- (static_cast<int64>(m_pp.copper_cursor) +
- (static_cast<int64>(m_pp.silver_cursor) * 10) +
- (static_cast<int64>(m_pp.gold_cursor) * 100) +
- (static_cast<int64>(m_pp.platinum_cursor) * 1000) +
- (static_cast<int64>(m_pp.platinum_shared) * 1000)))));
+ (static_cast<int64>(m_pp.copper)) +
+ (static_cast<int64>(m_pp.silver) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum) * PLATINUM_RATE) +
+ (static_cast<int64>(m_pp.copper_bank)) +
+ (static_cast<int64>(m_pp.silver_bank) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold_bank) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum_bank) * PLATINUM_RATE) +
+ (static_cast<int64>(m_pp.copper_cursor)) +
+ (static_cast<int64>(m_pp.silver_cursor) * SILVER_RATE) +
+ (static_cast<int64>(m_pp.gold_cursor) * GOLD_RATE) +
+ (static_cast<int64>(m_pp.platinum_cursor) * PLATINUM_RATE) +
+ (static_cast<int64>(m_pp.platinum_shared) * PLATINUM_RATE));
}
bool Client::CheckIncreaseSkill(SkillType skillid, Mob *against_who, int chancemodi) {
Index: client.h
===================================================================
--- client.h (revision 2229)
+++ client.h (working copy)
@@ -53,6 +53,11 @@
#define TARGETING_RANGE 200 // range for /assist and /target
#define XTARGET_HARDCAP 20
+#define PLATINUM_RATE 1000
+#define GOLD_RATE 100
+#define SILVER_RATE 10
+#define CONVERSION_RATE 10
+
extern Zone* zone;
extern TaskManager *taskmanager;
@@ -478,12 +483,20 @@
uint32 GetWeight() const { return(weight); }
inline void RecalcWeight() { weight = CalcCurrentWeight(); }
uint32 CalcCurrentWeight();
- inline uint32 GetCopper() const { return m_pp.copper; }
- inline uint32 GetSilver() const { return m_pp.silver; }
- inline uint32 GetGold() const { return m_pp.gold; }
- inline uint32 GetPlatinum() const { return m_pp.platinum; }
+ inline uint32 GetCopper() const { return m_pp.copper; }
+ inline uint32 GetSilver() const { return m_pp.silver; }
+ inline uint32 GetGold() const { return m_pp.gold; }
+ inline uint32 GetPlatinum() const { return m_pp.platinum; }
+ inline uint32 GetBankCopper() const { return m_pp.copper_bank; }
+ inline uint32 GetBankSilver() const { return m_pp.silver_bank; }
+ inline uint32 GetBankGold() const { return m_pp.gold_bank; }
+ inline uint32 GetBankPlatinum() const { return m_pp.platinum_bank; }
+ inline uint32 GetSharedPlatinum() const { return m_pp.platinum_shared; }
+ inline uint32 GetCursorCopper() const { return m_pp.copper_cursor; }
+ inline uint32 GetCursorSilver() const { return m_pp.silver_cursor; }
+ inline uint32 GetCursorGold() const { return m_pp.gold_cursor; }
+ inline uint32 GetCursorPlatinum() const { return m_pp.platinum_cursor; }
-
/*Endurance and such*/
void CalcMaxEndurance(); //This calculates the maximum endurance we can have
sint32 CalcBaseEndurance(); //Calculates Base End
@@ -619,6 +632,9 @@
void AddMoneyToPP(uint32 copper, uint32 silver, uint32 gold,uint32 platinum,bool updateclient);
bool HasMoney(uint64 copper);
int64 GetCarriedMoney();
+ int64 GetBankMoney();
+ int64 GetSharedBankMoney();
+ int64 GetCursorMoney();
int64 GetAllMoney();
bool IsDiscovered(int32 itemid);
Index: command.cpp
===================================================================
--- command.cpp (revision 2229)
+++ command.cpp (working copy)
@@ -263,7 +263,7 @@
command_add("appearance","[type] [value] - Send an appearance packet for you or your target",150,command_appearance) ||
command_add("charbackup","[list/restore] - Query or restore character backups",150,command_charbackup) ||
command_add("nukeitem","[itemid] - Remove itemid from your player target's inventory",150,command_nukeitem) ||
- command_add("peekinv","[worn/cursor/inv/bank/trade/trib/all] - Print out contents of your player target's inventory",100,command_peekinv) ||
+ command_add("peekinv","[money/worn/cursor/inv/bank/trade/trib/all] - Print out contents of your player target's inventory",100,command_peekinv) ||
command_add("findnpctype","[search criteria] - Search database NPC types",100,command_findnpctype) ||
command_add("findzone","[search criteria] - Search database zones",100,command_findzone) ||
command_add("fz",NULL,100,command_findzone) ||
@@ -3028,8 +3028,35 @@
bool bFound = false;
Client* client = c->GetTarget()->CastToClient();
const Item_Struct* item = NULL;
- c->Message(0, "Displaying inventory for %s...", client->GetName());
+ c->Message(15, "Displaying inventory for %s:", client->GetName());
+
+ if (bAll || (strcasecmp(sep->arg[1], "money")==0)) {
+ // Money
+ bFound = true;
+ int64 money_amt;
+ uint32 denom_amt;
+
+ money_amt=client->GetAllMoney(); c->Message((money_amt==0), "Total Money (in Copper): %i", money_amt);
+ money_amt=client->GetCarriedMoney(); c->Message((money_amt==0), "Carried Money (in Copper): %i", money_amt);
+ money_amt=client->GetBankMoney(); c->Message((money_amt==0), "Bank Money (in Copper): %i", money_amt);
+ money_amt=client->GetSharedBankMoney(); c->Message((money_amt==0), "Shared Bank Money (in Copper): %i", money_amt);
+ money_amt=client->GetCursorMoney(); c->Message((money_amt==0), "Cursor Money (in Copper): %i", money_amt);
+ denom_amt=client->GetPlatinum(); c->Message((denom_amt==0), "Carried Platinum: %i", denom_amt);
+ denom_amt=client->GetGold(); c->Message((denom_amt==0), "Carried Gold: %i", denom_amt);
+ denom_amt=client->GetSilver(); c->Message((denom_amt==0), "Carried Silver: %i", denom_amt);
+ denom_amt=client->GetCopper(); c->Message((denom_amt==0), "Carried Copper: %i", denom_amt);
+ denom_amt=client->GetBankPlatinum(); c->Message((denom_amt==0), "Bank Platinum: %i", denom_amt);
+ denom_amt=client->GetBankGold(); c->Message((denom_amt==0), "Bank Gold: %i", denom_amt);
+ denom_amt=client->GetBankSilver(); c->Message((denom_amt==0), "Bank Silver: %i", denom_amt);
+ denom_amt=client->GetBankCopper(); c->Message((denom_amt==0), "Bank Copper: %i", denom_amt);
+ denom_amt=client->GetSharedPlatinum(); c->Message((denom_amt==0), "Shared Bank Platinum: %i", denom_amt);
+ denom_amt=client->GetCursorPlatinum(); c->Message((denom_amt==0), "Cursor Platinum: %i", denom_amt);
+ denom_amt=client->GetCursorGold(); c->Message((denom_amt==0), "Cursor Gold: %i", denom_amt);
+ denom_amt=client->GetCursorSilver(); c->Message((denom_amt==0), "Cursor Silver: %i", denom_amt);
+ denom_amt=client->GetCursorCopper(); c->Message((denom_amt==0), "Cursor Copper: %i", denom_amt);
+ }
+
if (bAll || (strcasecmp(sep->arg[1], "worn")==0)) {
// Worn items
bFound = true;
@@ -3331,7 +3358,7 @@
if (!bFound)
{
- c->Message(0, "Usage: #peekinv [worn|cursor|inv|bank|trade|trib|all]");
+ c->Message(0, "Usage: #peekinv [money|worn|cursor|inv|bank|trade|trib|all]");
c->Message(0, " Displays a portion of the targeted user's inventory");
c->Message(0, " Caution: 'all' is a lot of information!");
}
__________________
Uleat of Bertoxxulous
Compilin' Dirty
|
 |
|
 |

10-18-2012, 11:30 PM
|
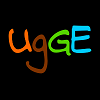 |
Developer
|
|
Join Date: Apr 2012
Location: North Carolina
Posts: 2,815
|
|
It never fails... When I submitted the bandolier patch, three weeks went by and I had no negative feedback. The day after I reformatted my system, a problem
crept up.
The day after I submitted this patch, my hard drive failed... I do have an updated version of this that is a little more efficient..but, regardless, I won't be
able to support this patch until I can get a new hard drive and get my system back up to speed.
I thought that I was having some sort of conflict with tortoise and that was my reason for reformatting, but I guess it was the first sign of my drive going...
Use this patch at your own risk for the time being as I won't be able to troubleshoot issues until my system is fixed.
Sorry about that guys (and gals!)
__________________
Uleat of Bertoxxulous
Compilin' Dirty
|

10-19-2012, 01:52 AM
|
Discordant
|
|
Join Date: Dec 2005
Posts: 435
|
|
Ah that sucks man. Hope you get your stuff back up soon.
|
 |
|
 |

10-23-2012, 09:31 PM
|
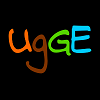 |
Developer
|
|
Join Date: Apr 2012
Location: North Carolina
Posts: 2,815
|
|
I did get it back up, thanks! Definitely a bad drive... Having other issues now though O.o
I need to do a better job of separating bug fixes from alternative code. If someone wants to bump this thread up to 'Custom Code,' please do.
Anyways... Here is the fully implented and focused '#peekinv money' patch that should be what's posted here.
[PeekMoney.patch]
Code:
Index: client.cpp
===================================================================
--- client.cpp (revision 2241)
+++ client.cpp (working copy)
@@ -2229,28 +2229,53 @@
int64 Client::GetCarriedMoney() {
- return ((static_cast<int64>(m_pp.copper) +
+ return (
+ (static_cast<int64>(m_pp.copper)) +
(static_cast<int64>(m_pp.silver) * 10) +
(static_cast<int64>(m_pp.gold) * 100) +
- (static_cast<int64>(m_pp.platinum) * 1000)));
+ (static_cast<int64>(m_pp.platinum) * 1000));
}
+int64 Client::GetBankMoney() {
+
+ return (
+ (static_cast<int64>(m_pp.copper_bank)) +
+ (static_cast<int64>(m_pp.silver_bank) * 10) +
+ (static_cast<int64>(m_pp.gold_bank) * 100) +
+ (static_cast<int64>(m_pp.platinum_bank) * 1000));
+}
+
+int64 Client::GetSharedBankMoney() {
+
+ return (
+ (static_cast<int64>(m_pp.platinum_shared) * 1000));
+}
+
+int64 Client::GetCursorMoney() {
+
+ return (
+ (static_cast<int64>(m_pp.copper_cursor)) +
+ (static_cast<int64>(m_pp.silver_cursor) * 10) +
+ (static_cast<int64>(m_pp.gold_cursor) * 100) +
+ (static_cast<int64>(m_pp.platinum_cursor) * 1000));
+}
+
int64 Client::GetAllMoney() {
return (
- (static_cast<int64>(m_pp.copper) +
+ (static_cast<int64>(m_pp.copper)) +
(static_cast<int64>(m_pp.silver) * 10) +
(static_cast<int64>(m_pp.gold) * 100) +
(static_cast<int64>(m_pp.platinum) * 1000) +
- (static_cast<int64>(m_pp.copper_bank) +
+ (static_cast<int64>(m_pp.copper_bank)) +
(static_cast<int64>(m_pp.silver_bank) * 10) +
(static_cast<int64>(m_pp.gold_bank) * 100) +
(static_cast<int64>(m_pp.platinum_bank) * 1000) +
- (static_cast<int64>(m_pp.copper_cursor) +
+ (static_cast<int64>(m_pp.copper_cursor)) +
(static_cast<int64>(m_pp.silver_cursor) * 10) +
(static_cast<int64>(m_pp.gold_cursor) * 100) +
(static_cast<int64>(m_pp.platinum_cursor) * 1000) +
- (static_cast<int64>(m_pp.platinum_shared) * 1000)))));
+ (static_cast<int64>(m_pp.platinum_shared) * 1000));
}
bool Client::CheckIncreaseSkill(SkillType skillid, Mob *against_who, int chancemodi) {
Index: client.h
===================================================================
--- client.h (revision 2241)
+++ client.h (working copy)
@@ -478,12 +478,20 @@
uint32 GetWeight() const { return(weight); }
inline void RecalcWeight() { weight = CalcCurrentWeight(); }
uint32 CalcCurrentWeight();
- inline uint32 GetCopper() const { return m_pp.copper; }
- inline uint32 GetSilver() const { return m_pp.silver; }
- inline uint32 GetGold() const { return m_pp.gold; }
- inline uint32 GetPlatinum() const { return m_pp.platinum; }
+ inline uint32 GetCopper() const { return m_pp.copper; }
+ inline uint32 GetSilver() const { return m_pp.silver; }
+ inline uint32 GetGold() const { return m_pp.gold; }
+ inline uint32 GetPlatinum() const { return m_pp.platinum; }
+ inline uint32 GetBankCopper() const { return m_pp.copper_bank; }
+ inline uint32 GetBankSilver() const { return m_pp.silver_bank; }
+ inline uint32 GetBankGold() const { return m_pp.gold_bank; }
+ inline uint32 GetBankPlatinum() const { return m_pp.platinum_bank; }
+ inline uint32 GetSharedPlatinum() const { return m_pp.platinum_shared; }
+ inline uint32 GetCursorCopper() const { return m_pp.copper_cursor; }
+ inline uint32 GetCursorSilver() const { return m_pp.silver_cursor; }
+ inline uint32 GetCursorGold() const { return m_pp.gold_cursor; }
+ inline uint32 GetCursorPlatinum() const { return m_pp.platinum_cursor; }
-
/*Endurance and such*/
void CalcMaxEndurance(); //This calculates the maximum endurance we can have
sint32 CalcBaseEndurance(); //Calculates Base End
@@ -619,6 +627,9 @@
void AddMoneyToPP(uint32 copper, uint32 silver, uint32 gold,uint32 platinum,bool updateclient);
bool HasMoney(uint64 copper);
int64 GetCarriedMoney();
+ int64 GetBankMoney();
+ int64 GetSharedBankMoney();
+ int64 GetCursorMoney();
int64 GetAllMoney();
bool IsDiscovered(int32 itemid);
Index: command.cpp
===================================================================
--- command.cpp (revision 2241)
+++ command.cpp (working copy)
@@ -263,7 +263,7 @@
command_add("appearance","[type] [value] - Send an appearance packet for you or your target",150,command_appearance) ||
command_add("charbackup","[list/restore] - Query or restore character backups",150,command_charbackup) ||
command_add("nukeitem","[itemid] - Remove itemid from your player target's inventory",150,command_nukeitem) ||
- command_add("peekinv","[worn/cursor/inv/bank/trade/trib/all] - Print out contents of your player target's inventory",100,command_peekinv) ||
+ command_add("peekinv","[money/worn/cursor/inv/bank/trade/trib/all] - Print out contents of your player target's inventory",100,command_peekinv) ||
command_add("findnpctype","[search criteria] - Search database NPC types",100,command_findnpctype) ||
command_add("findzone","[search criteria] - Search database zones",100,command_findzone) ||
command_add("fz",NULL,100,command_findzone) ||
@@ -3028,8 +3028,38 @@
bool bFound = false;
Client* client = c->GetTarget()->CastToClient();
const Item_Struct* item = NULL;
- c->Message(0, "Displaying inventory for %s...", client->GetName());
+ c->Message(15, "Displaying inventory for %s:", client->GetName());
+ if (bAll || (strcasecmp(sep->arg[1], "money")==0)) {
+ // Money
+ bFound = true;
+ int64 money_amt;
+ uint32 denom_amt;
+
+ money_amt=client->GetAllMoney(); c->Message((money_amt==0), "TOTAL MONEY (in Copper): %i", money_amt);
+
+ money_amt=client->GetCarriedMoney(); c->Message((money_amt==0), "Carried Money (in Copper): %i", money_amt);
+ denom_amt=client->GetPlatinum(); c->Message((denom_amt==0), "---Carried Platinum: %i", denom_amt);
+ denom_amt=client->GetGold(); c->Message((denom_amt==0), "---Carried Gold: %i", denom_amt);
+ denom_amt=client->GetSilver(); c->Message((denom_amt==0), "---Carried Silver: %i", denom_amt);
+ denom_amt=client->GetCopper(); c->Message((denom_amt==0), "---Carried Copper: %i", denom_amt);
+
+ money_amt=client->GetBankMoney(); c->Message((money_amt==0), "Bank Money (in Copper): %i", money_amt);
+ denom_amt=client->GetBankPlatinum(); c->Message((denom_amt==0), "---Bank Platinum: %i", denom_amt);
+ denom_amt=client->GetBankGold(); c->Message((denom_amt==0), "---Bank Gold: %i", denom_amt);
+ denom_amt=client->GetBankSilver(); c->Message((denom_amt==0), "---Bank Silver: %i", denom_amt);
+ denom_amt=client->GetBankCopper(); c->Message((denom_amt==0), "---Bank Copper: %i", denom_amt);
+
+ money_amt=client->GetSharedBankMoney(); c->Message((money_amt==0), "Shared Bank Money (in Copper): %i", money_amt);
+ denom_amt=client->GetSharedPlatinum(); c->Message((denom_amt==0), "---Shared Bank Platinum: %i", denom_amt);
+
+ money_amt=client->GetCursorMoney(); c->Message((money_amt==0), "Cursor Money (in Copper): %i", money_amt);
+ denom_amt=client->GetCursorPlatinum(); c->Message((denom_amt==0), "---Cursor Platinum: %i", denom_amt);
+ denom_amt=client->GetCursorGold(); c->Message((denom_amt==0), "---Cursor Gold: %i", denom_amt);
+ denom_amt=client->GetCursorSilver(); c->Message((denom_amt==0), "---Cursor Silver: %i", denom_amt);
+ denom_amt=client->GetCursorCopper(); c->Message((denom_amt==0), "---Cursor Copper: %i", denom_amt);
+ }
+
if (bAll || (strcasecmp(sep->arg[1], "worn")==0)) {
// Worn items
bFound = true;
@@ -3038,14 +3068,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "WornSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "WornSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "WornSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "WornSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3060,14 +3090,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "InvSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "InvSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "InvSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "InvSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3079,7 +3109,7 @@
item = (instbag) ? instbag->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), " InvBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---InvBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3087,7 +3117,7 @@
}
else
{
- c->Message((item==0), " InvBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---InvBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3100,7 +3130,7 @@
{
const ItemInst* inst = client->GetInv().GetItem(9999);
item = (inst) ? inst->GetItem() : NULL;
- c->Message((item==0), "InvSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", 9999,
+ c->Message((item==0), "InvSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", 9999,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3118,12 +3148,12 @@
if(client->GetInv().CursorEmpty()) { // Display 'front' cursor slot even if 'empty' (item(30[0]) == null)
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", SLOT_CURSOR,i,
+ c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", SLOT_CURSOR,i,
0, 0x12, 0, "null", 0x12, 0);
}
else
{
- c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", SLOT_CURSOR,i,
+ c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", SLOT_CURSOR,i,
0, 0x12, 0, "null", 0x12, 0);
}
}
@@ -3133,14 +3163,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", SLOT_CURSOR,i,
+ c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", SLOT_CURSOR,i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", SLOT_CURSOR,i,
+ c->Message((item==0), "CursorSlot: %i, Depth: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", SLOT_CURSOR,i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3152,7 +3182,7 @@
item = (instbag) ? instbag->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), " CursorBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---CursorBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(SLOT_CURSOR, j),
SLOT_CURSOR, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3160,7 +3190,7 @@
}
else
{
- c->Message((item==0), " CursorBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---CursorBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(SLOT_CURSOR, j),
SLOT_CURSOR, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3180,14 +3210,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "TributeSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "TributeSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "TributeSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "TributeSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3204,14 +3234,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "BankSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "BankSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "BankSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "BankSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3223,7 +3253,7 @@
item = (instbag) ? instbag->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), " BankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---BankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3231,7 +3261,7 @@
}
else
{
- c->Message((item==0), " BankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---BankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3245,14 +3275,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "ShBankSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "ShBankSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "ShBankSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "ShBankSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3264,7 +3294,7 @@
item = (instbag) ? instbag->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), " ShBankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---ShBankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3272,7 +3302,7 @@
}
else
{
- c->Message((item==0), " ShBankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---ShBankBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3290,14 +3320,14 @@
item = (inst) ? inst->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), "TradeSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "TradeSlot: %i, Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
}
else
{
- c->Message((item==0), "TradeSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i", i,
+ c->Message((item==0), "TradeSlot: %i, Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i", i,
((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
((item==0)?0:inst->GetCharges()));
@@ -3309,7 +3339,7 @@
item = (instbag) ? instbag->GetItem() : NULL;
if (c->GetClientVersion() >= EQClientSoF)
{
- c->Message((item==0), " TradeBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---TradeBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X00000000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3317,7 +3347,7 @@
}
else
{
- c->Message((item==0), " TradeBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Charges: %i",
+ c->Message((item==0), "---TradeBagSlot: %i (Slot #%i, Bag #%i), Item: %i (%c%06X000000000000000000000000000000000000000%s%c), Count: %i",
Inventory::CalcSlotId(i, j),
i, j, ((item==0)?0:item->ID),0x12, ((item==0)?0:item->ID),
((item==0)?"null":item->Name), 0x12,
@@ -3331,7 +3361,7 @@
if (!bFound)
{
- c->Message(0, "Usage: #peekinv [worn|cursor|inv|bank|trade|trib|all]");
+ c->Message(0, "Usage: #peekinv [money|worn|cursor|inv|bank|trade|trib|all]");
c->Message(0, " Displays a portion of the targeted user's inventory");
c->Message(0, " Caution: 'all' is a lot of information!");
}
__________________
Uleat of Bertoxxulous
Compilin' Dirty
|
 |
|
 |
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 08:56 AM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |