|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
Development::Development Forum for development topics and for those interested in EQEMu development. (Not a support forum) |
 |
|
 |

04-02-2012, 07:56 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Mercenaries
Since EQ is now F2P, I spent some time collecting data from Live for Mercenaries. There does not seem to be too many packets related to Mercs. Below is what I have figured out for mercenary packet structures. Hopefully I can get time to test some of this and maybe implement some basic handling for these packets, but I figured it would be good to post in case I don't get time to do that soon.
Mercenary Packet Structures:
Code:
// Currently unused, but may be good to have
enum MercStance
{
MercPassive = 1,
MercBalanced = 2,
MercEfficient = 3,
MercReactive = 4,
MercAggressive = 5,
MercAssist = 6,
MercBurn = 7,
MercEfficient = 8,
MercBurnAE = 9
};
// [OPCode: 0x27ac] On Live as of April 2 2012 [Server->Client]
struct MercenarySellList_Struct {
int32 MercTypeCount; // Seen 2 - Iterations of MercCategory_Struct
MercCategory_Struct Categories[0]; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
int32 MercCount; // Seen 24 - Iterations of MercCategory_Struct
MercenaryInfo_Struct Mercs[0]; // Data for individual mercenaries in the Merchant List
};
struct MercCategory_Struct {
int32 Category; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
};
// Used for Mercenary Merchant Lists and for Hired Mercenary Info Updates
struct MercenaryInfo_Struct {
int32 MercID; // ID unique to each type of mercenary (probably a DB id) - (if 1, do not send MercData_Struct - No merc hired)
MercData_Struct MercData[0]; // Data for individual mercenaries - Not populated if no merc is hired
};
struct MercData_Struct {
/*0000*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0004*/ int32 MercDesc; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0008*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0012*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0016*/ int32 Status; // Required Account Status (Free = 0, Silver = 1, Gold = 2) at merchants - Seen 0 (suspended) or 1 (unsuspended) on hired mercs ?
/*0020*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0024*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0028*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0032*/ int8 MercUnk01; // Unknown (always see 0)
/*0036*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0040*/ int32 MerchantSlot; // Merchant Slot? Increments, but not always by 1 - May be for Merc Window Options (Seen 5, 36, 1 for active mercs)?
/*0044*/ int32 MercUnk02; // Unknown (always see 1)
/*0048*/ int32 StanceCount; // Iterations of MercStance_Struct - Normally 2 to 4 seen
/*0052*/ int32 MercUnk03; // Unknown (always 0 at merchant) - Seen on active merc: 93 a4 03 77, b8 ed 2f 26, 88 d5 8b c3, and 93 a4 ad 77
/*0056*/ int8 MercUnk04; // Seen 1
/*0060*/ char[0] MercName; // Null Terminated Mercenary Name (00 at merchants)
/*0000*/ MercStance_Struct Stances[0]; // From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
};
// There appears to be extra data sent in this part of the struct sometimes that is unknown (normally 0, 1, or 2)
struct MercStance_Struct {
int32 Stance; // From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
};
// [OPCode: 0x6537] On Live as of April 2 2012 [Server->Client]
// Size 12 and sent on Zone-In if no mercenary is currently hired and when merc is dismissed
// Size varies if mercenary is hired or if browsing Mercenary Merchant
struct MercDataUpdate_Struct {
/*0000*/ sint32 MercStatus; // Seen 0 with merc and -1 with no merc hired
/*0004*/ int32 HiredCount; // Seen 1 with 1 merc hired and 0 with no merc hired
/*0008*/ MercenaryInfo_Struct MercData[0]; // Data for individual mercenaries in the Merchant List
};
// [OPCode: 0x495d] On Live as of April 2 2012 [Server->Client] [Size: 20]
// Sent on Zone-In, or after Dismissing, Suspending or Unsuspending Mercs
struct MercenaryUpdate_Struct {
/*0000*/ int32 MercEntityID; // Seen 0 (no merc spawned) or 615843841 and 22779137
/*0004*/ int32 UpdateInterval; // Seen 900000 - Matches from 0x6537 packet (15 minutes in ms?)
/*0008*/ int32 MercUnk01; // Seen 180000 - 3 minutes in milleseconds?
/*0012*/ int32 MercState; // Seen 5 (normal) or 1 (suspended)
/*0016*/ int32 SuspendedTime; // Seen 0 (not suspended) or c9 c2 64 4f (suspended on Sat Mar 17 11:58:49 2012) - Unix Timestamp
/*0020*/
};
// [OPCode: 0x1a79] On Live as of April 2 2012 [Client->Server] [Size: 1] - Requesting to suspend or unsuspend merc
struct SuspendMercenary_Struct {
/*0000*/ int8 SuspendMerc; // Seen 30 (48) for suspending or unsuspending
/*0001*/
};
// [OPCode: 0x2528] On Live as of April 2 2012 [Server->Client] [Size: 4] - Response to suspend merc with timestamp
struct SuspendMercenary_Struct {
/*0000*/ int32 SuspendTime; // Unix Timestamp - Seen a9 11 78 4f
/*0004*/
};
// MercCommand field is possibly related to MerchantSlot field in MercData_Struct (seen same values)
// [OPCode: 0x4c6c] On Live as of April 2 2012 [Client->Server] [Size: 8] - Response to suspend merc with timestamp
struct MercCommand_Struct {
/*0000*/ int32 MercCommand; // Seen 0 (zone in with no merc or suspended), 1 (dismiss merc), 5 (normal state), 36 (zone in with merc)
/*0004*/ int32 Option; // Seen -1 (zone in with no merc), 0 (setting to passive stance), 1 (normal or setting to balanced stance)
/*0008*/
};
These are the opcodes that appear to be directly Mercenary related:
0x6537 Server->Client - Some kinda merc info update. Much smaller with no merc
0x0327 Client->Server - Labelled OP_MercenaryDataResponse in EQExtractor2 (size 0)
0x2ef8 Client->Server - Request dismiss merc (size 0)
0x495d Server->Client - Some merc status update from server - Maybe response to 0x4c6c
0x1a79 Client->Server - Requesting to suspend merc
0x2528 Server->Client - Response to suspend merc
0x4c6c Client->Server - Some kind of merc status change request from client - Examples:
24 00 00 00 01 00 00 00 - Zoning in
01 00 00 00 01 00 00 00 - Dismiss merc
05 00 00 00 01 00 00 00 - Right after purchasing merc
05 00 00 00 00 00 00 00 - Setting merc to passive
05 00 00 00 01 00 00 00 - Setting merc back to balanced
00 00 00 00 ff ff ff ff - Zoning in with no merc
01 00 00 00 01 00 00 00 - Right after previous update of zoning in with no merc
Using Mercenary Merchant:
0x4dd9 Client->Server - Right clicking merchant request
0x27ac Server->Client - Merc merchant response
0x3887 Server->Client - After merchant response
0x5e78 Client->Server - Request to view merc info while browsing merchant?
90 01 00 00 01 00 00 00 46 22 00 00 00 6e e4 03
0x5e78 Server->Client - Server response to 0x5e78
06 00 00 00
0x528f Server->Client - Seen after purchasing merc and when camping
0x6942 Server->Client - Response after purchasing merc - Purchase approval response?
I have a ton more data than I am showing here, but I don't want to post it all until I can organize it a bit more. I will post the rest of the structs for the above lists when I have time. At least the more complex structs should be in pretty good shape from the ones I posted above. The rest are all really small (16 bytes or less).
|
 |
|
 |

04-02-2012, 10:29 PM
|
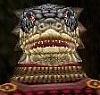 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Quote:
Originally Posted by trevius
*snip*
|
This is actually going to help me a lot, I had mercenaries figured out to the point where I could actually have the window up, but I wasn't going off of live packet collects. I'll look into finding out what some of those unknowns are for you.
Last edited by Secrets; 04-02-2012 at 11:18 PM..
|
 |
|
 |

04-02-2012, 10:53 PM
|
Developer
|
|
Join Date: Feb 2009
Location: Cincinnati, OH
Posts: 512
|
|
Trev, I have a lot of this worked out for SoD and UF, with the help of Derision. We are able to display the info in the merc merchant's window, and can spawn an npc that uses the merc window, changing stances, etc. I spent a lot of time getting all of the data (I generated quite a few hundred inserts for a basic db structure for mercs for types, subtypes, stances, handling different clients, and started on inventory and spells) for the freely available mercs before free to play which further restricted to just apprentice mercs (was apprentice t1-5 and journeymen 1-2).
If you plan on working on mercs, I can send all of it to you, or if you are just getting the info out there, I can use anything you have. I was trying to get to mercs within a few weeks. I've been really busy lately though.
Things have changed recently with the ability to have multiple mercs and such, but the basics should still be the same. I am on live trying to lvl up my toons to have a full group with mercs so I can continue to get spells cast as well as screenshots of equipment, etc. Let me know if there's anything you need.
|
 |
|
 |
 |
|
 |

04-03-2012, 12:51 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Most of the data I have is related to what I already posted. Just the basic purchasing and usage packets and structs from Live. It sounds like you guys are a lot further along than I am lol. I only really worked on it for 1 day though. I didn't know anyone else was working on it. With your recent implementation of stances, I figured it would make it that much easier to add in a merc system.
I am a bit interested in the DB work you have done for them. I didn't think it would take hundreds of entries for it. I was figuring on 1 entry per merc subtype in a new merc table that just points to an NPC ID in the npc_types table. I haven't really played Live since 2005 until now (since it went free), so I am still really new to mercs. I guess there would be a lot of variations for merc races, and multiple types and subtypes.
I am not really too concerned with the DB entries, as I would probably just add somewhat customized entries anyway. I was more looking to get some basic functionality implemented that could be built upon. It sounds like you guys have already made it that far and are working on finishing stuff off.
I am no where near as skilled as Derision or you when it comes to that type of thing, so I will leave it up to the 2 of you
Maybe I can help you guys out if there is something you still need done. I am better with dissecting packets than I am with coding normally. I have quite a few characters in the 50 to 67 range, if there is something I can get with them that would be useful.
I can post the rest of the smaller structs later tonight (I still have to make them) if they would be useful. I also plan to write a script to help with opcode work a bit hopefully.
You should join the dev IRC channel. It might be easier to discuss and share info there. PM me if you don't know the channel name.
|
 |
|
 |

09-20-2012, 06:39 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Bad_captain, did you ever get anywhere with this? I am curious how close this was to being functional. Even if it is only partly functional, it might be good to get it on the SVN so the rest can be completed as time permits. I could probably put some more work in on it, but didn't want to double any efforts that have already been made.
|

09-20-2012, 08:06 AM
|
Opcode Ninja
|
|
Join Date: Mar 2009
Location: San francisco
Posts: 426
|
|
i'll post the newer opcodes real soon
Code:
Listing of hired mercenaries
OP_MercenaryList=0x5a0a
|

09-21-2012, 12:53 AM
|
Developer
|
|
Join Date: Feb 2009
Location: Cincinnati, OH
Posts: 512
|
|
Sorry I dropped the ball on this one. I have meant to get back to this, but keep finding stuff to fix in bots, and with school just starting back up, I've been pretty busy. I'm in the middle of a bot AA rewrite, then I plan on getting back to this. I know I was slowed down working on all of the packet encoding/decoding, which I know you are adept at. If I can't get something going by next weekend (hopefully I will have time to at least get it somewhat respectable), I'll shoot you a diff or something.
I have been trying to level up on live to get some better logs/collects, but it's been slow going. I've been getting screenshots though, as weapons appear to change every 5 levels (not that I think we can get all equipment correct, but it's at least a start).
|
 |
|
 |

10-12-2012, 06:15 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
I went through and refined, corrected, and added to the previous list of Mercenary related packet structures that I had posted. Each includes the opcode used on Live as well as the opcode name used on EQEmu currently where I could find them. I added notes for each struct to explain the packet a bit.
This should compile find on windows now, and should work for the initial changes to get mercs to be purchaseable and spawning at least. These structs are the ones used on Live as of April 12 2012, so these would go in eq_packet_structs.h. I will go through the SoD and UF clients and add the structs for those patch files as well in the next post.
Right now, there are still a couple of struct issues remaining due to how the variable sized packets for the Mercenary Data has variable sized arrays within it. To prevent it from breaking anything, I just hard set the variable sized structs that are inside the already variable sized structs. This only effects a few fields, but it is something that will need to be corrected to make these packets work exactly like they do on Live. For example, the MercName, MercTypes,and Stances fields are all hard set to values that should make them functional enough for now, but ideally those should all be able to vary in size. I am not really sure of the best way to do this and still allow the data to be sent to patch .cpp files so they can be encoded accordingly for each client.
Code:
// Used by MercenaryListEntry_Struct
struct MercenaryStance_Struct {
/*0000*/ int32 StanceIndex; // Index of this stance (sometimes reverse reverse order - 3, 2, 1, 0 for 4 stances etc)
/*0004*/ int32 Stance; // From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
};
// Used by MercenaryMerchantList_Struct
struct MercenaryListEntry_Struct {
/*0000*/ int32 MercID; // ID unique to each type of mercenary (probably a DB id)
/*0004*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0008*/ int32 MercSubType; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0012*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0016*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0020*/ int32 Status; // Required Account Status (Free = 0, Silver = 1, Gold = 2) at merchants - Seen 0 (suspended) or 1 (unsuspended) on hired mercs ?
/*0024*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0028*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0032*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0036*/ int8 MercUnk01; // Unknown (always see 0)
/*0037*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0041*/ int32 MerchantSlot; // Merchant Slot? Increments, but not always by 1 - May be for Merc Window Options (Seen 5, 36, 1 for active mercs)?
/*0045*/ int32 MercUnk02; // Unknown (normally see 1, but sometimes 2 or 0)
/*0049*/ int32 StanceCount; // Iterations of MercenaryStance_Struct - Normally 2 to 4 seen
/*0053*/ int32 MercUnk03; // Unknown (always 0 at merchant) - Seen on active merc: 93 a4 03 77, b8 ed 2f 26, 88 d5 8b c3, and 93 a4 ad 77
/*0057*/ int8 MercUnk04; // Seen 1
/*0058*/ char MercName[1]; // Null Terminated Mercenary Name (00 at merchants)
/*0000*/ MercenaryStance_Struct Stances[2]; // Count Varies, but hard set to 2 for now - From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
};
// [OPCode: 0x27ac OP_MercenaryDataResponse] On Live as of April 2 2012 [Server->Client]
// Opcode should be renamed to something like OP_MercenaryMerchantShopResponse since the Data Response packet is different
// Sent by the server when browsing the Mercenary Merchant
struct MercenaryMerchantList_Struct {
/*0000*/ int32 MercTypeCount; // Number of Merc Types to follow
/*0004*/ int32 MercTypes[3]; // Count varies, but hard set to 3 for now - From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0016*/ int32 MercCount; // Number of MercenaryInfo_Struct to follow
/*0020*/ MercenaryListEntry_Struct Mercs[0]; // Data for individual mercenaries in the Merchant List
};
// [OPCode: 0x4dd9 OP_MercenaryDataRequest] On Live as of April 2 2012 [Client->Server]
// Opcode should be renamed to something like OP_MercenaryMerchantShopRequest since the Data Request packet is different
// Right clicking merchant - shop request
struct MercenaryMerchantShopRequest_Struct {
/*0000*/ int32 MercMerchantID; // Entity ID of the Mercenary Merchant
/*0004*/
};
// Used by MercenaryDataUpdate_Struct
struct MercenaryData_Struct {
/*0000*/ int32 MercID; // ID unique to each type of mercenary (probably a DB id) - (if 1, do not send MercenaryData_Struct - No merc hired)
/*0004*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0008*/ int32 MercSubType; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0012*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0016*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0020*/ int32 Status; // Required Account Status (Free = 0, Silver = 1, Gold = 2) at merchants - Seen 0 (suspended) or 1 (unsuspended) on hired mercs ?
/*0024*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0028*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0032*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0036*/ int8 MercUnk01; // Unknown (always see 0)
/*0037*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0041*/ int32 MerchantSlot; // Merchant Slot? Increments, but not always by 1 - May be for Merc Window Options (Seen 5, 36, 1 for active mercs)?
/*0045*/ int32 MercUnk02; // Unknown (normally see 1, but sometimes 2 or 0)
/*0049*/ int32 StanceCount; // Iterations of MercenaryStance_Struct - Normally 2 to 4 seen
/*0053*/ int32 MercUnk03; // Unknown (always 0 at merchant) - Seen on active merc: 93 a4 03 77, b8 ed 2f 26, 88 d5 8b c3, and 93 a4 ad 77
/*0057*/ int8 MercUnk04; // Seen 1
/*0058*/ char MercName[1]; // Null Terminated Mercenary Name (00 at merchants)
/*0000*/ MercenaryStance_Struct Stances[2]; // Count Varies, but hard set to 2 for now - From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
/*0000*/ int32 MercUnk05; // Seen 1 - Extra Merc Data field that differs from MercenaryListEntry_Struct
// MercUnk05 may be a field that is at the end of the packet only, even if multiple mercs are listed (haven't seen examples of multiple mercs owned at once)
};
// [OPCode: 0x6537] On Live as of April 2 2012 [Server->Client]
// Should be named OP_MercenaryDataResponse, but the current opcode using that name should be renamed first
// Size varies if mercenary is hired or if browsing Mercenary Merchant
// This may also be the response for Client->Server 0x0327 (size 0) packet On Live as of April 2 2012
struct MercenaryDataUpdate_Struct {
/*0000*/ sint32 MercStatus; // Seen 0 with merc and -1 with no merc hired
/*0004*/ int32 MercCount; // Seen 1 with 1 merc hired and 0 with no merc hired
/*0008*/ MercenaryData_Struct MercData[0]; // Data for individual mercenaries in the Merchant List
};
// [OPCode: 0x6537] On Live as of April 2 2012 [Server->Client]
// Size 12 and sent on Zone-In if no mercenary is currently hired and when merc is dismissed
// (Same packet as MercAssign_Struct?)
struct NoMercenaryHired_Struct {
/*0000*/ sint32 MercStatus; // Seen -1 with no merc hired
/*0004*/ int32 MercCount; // Seen 0 with no merc hired
/*0008*/ int32 MercID; // Seen 1 when no merc is hired - ID unique to each type of mercenary
/*0012*/
};
// OP_MercenaryAssign (Same packet as NoMercenaryHired_Struct?)
struct MercenaryAssign_Struct {
/*0000*/ int32 MercEntityID; // Seen 0 (no merc spawned) or 615843841 and 22779137
/*0004*/ int32 MercUnk01; //
/*0008*/ int32 MercUnk02; //
/*0012*/
};
// [OPCode: 0x495d OP_MercenaryTimer] On Live as of April 2 2012 [Server->Client] [Size: 20]
// Sent on Zone-In, or after Dismissing, Suspending, or Unsuspending Mercs
struct MercenaryStatus_Struct {
/*0000*/ int32 MercEntityID; // Seen 0 (no merc spawned) or 615843841 and 22779137
/*0004*/ int32 UpdateInterval; // Seen 900000 - Matches from 0x6537 packet (15 minutes in ms?)
/*0008*/ int32 MercUnk01; // Seen 180000 - 3 minutes in milleseconds? Maybe next update interval?
/*0012*/ int32 MercState; // Seen 5 (normal) or 1 (suspended)
/*0016*/ int32 SuspendedTime; // Seen 0 (not suspended) or c9 c2 64 4f (suspended on Sat Mar 17 11:58:49 2012) - Unix Timestamp
/*0020*/
};
// [OPCode: 0x4c6c] On Live as of April 2 2012 [Client->Server] [Size: 8]
// Sent from the client when using the Mercenary Window
struct MercenaryCommand_Struct {
/*0000*/ int32 MercCommand; // Seen 0 (zone in with no merc or suspended), 1 (dismiss merc), 5 (normal state), 36 (zone in with merc)
/*0004*/ sint32 Option; // Seen -1 (zone in with no merc), 0 (setting to passive stance), 1 (normal or setting to balanced stance)
/*0008*/
};
// [OPCode: 0x1a79] On Live as of April 2 2012 [Client->Server] [Size: 1]
// Requesting to suspend or unsuspend merc
struct SuspendMercenary_Struct {
/*0000*/ int8 SuspendMerc; // Seen 30 (48) for suspending or unsuspending
/*0001*/
};
// [OPCode: 0x2528] On Live as of April 2 2012 [Server->Client] [Size: 4]
// Response to suspend merc with timestamp
struct SuspendMercenaryResponse_Struct {
/*0000*/ int32 SuspendTime; // Unix Timestamp - Seen a9 11 78 4f
/*0004*/
};
// [OPCode: 0x5e78 (OP_MercenaryHire?)] On Live as of April 2 2012
// Sent by client when requesting to view Mercenary info or Hire a Mercenary
struct MercenaryMerchantRequest_Struct {
/*0000*/ int32 MercID; // Seen 399 and 400 for merc ID
/*0004*/ int32 MercUnk01; // Seen 1
/*0008*/ int32 MercMerchantID; // Entity ID for Mercenary Merchant
/*0012*/ int32 MercUnk02; // Seen 65302016 (00 6e e4 03) - (probably actually individual int8 fields)
/*0016*/
};
// [OPCode: 0x5e78 (OP_MercenaryHire?)] On Live as of April 2 2012
// Sent by Server in response to requesting to view Mercenary info or Hire a Mercenary
struct MercenaryMerchantResponse_Struct {
/*0000*/ int32 ResponseType; // Seen 0 for hire response, 6 for info response, and 9 for denied hire request
/*0004*/
};
Last edited by trevius; 10-13-2012 at 08:49 AM..
|
 |
|
 |
 |
|
 |

10-12-2012, 07:26 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Here are the only struct differences for UF and SoD that I know so far based on the packets bad_captain and Derision were generating for each client:
SoD
Code:
// Used by MercenaryMerchantList_Struct
struct MercenaryListEntry_Struct {
/*0000*/ int32 MercID; // ID unique to each type of mercenary (probably a DB id)
/*0004*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0008*/ int32 MercSubType; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0012*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0016*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0020*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0024*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0028*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0032*/ int32 StanceCount; // Iterations of MercenaryStance_Struct - Normally 2 to 4 seen
/*0036*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0040*/ MercenaryStance_Struct Stances[2]; // Count Varies, but hard set to 2 for now - From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
};
// Used by MercenaryDataUpdate_Struct
struct MercenaryData_Struct {
/*0000*/ int32 MercID; // ID unique to each type of mercenary (probably a DB id)
/*0004*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0008*/ int32 MercSubType; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0012*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0016*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0020*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0024*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0028*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0032*/ int32 StanceCount; // Iterations of MercenaryStance_Struct - Normally 2 to 4 seen
/*0036*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0040*/ MercenaryStance_Struct Stances[2]; // Count Varies, but hard set to 2 for now - From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
/*0000*/ int32 MercUnk05; // Seen 1 - Extra Merc Data field that differs from MercenaryListEntry_Struct
// MercUnk05 may be a field that is at the end of the packet only, even if multiple mercs are listed (haven't seen examples of multiple mercs owned at once)
};
Underfoot
Code:
// Used by MercenaryMerchantList_Struct
struct MercenaryListEntry_Struct {
/*0000*/ int32 MercID; // ID unique to each type of mercenary (probably a DB id)
/*0004*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0008*/ int32 MercSubType; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0012*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0016*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0020*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0024*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0028*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0032*/ int8 MercUnk01; // Unknown (always see 0)
/*0033*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0037*/ int32 MerchantSlot; // Merchant Slot? Increments, but not always by 1 - May be for Merc Window Options (Seen 5, 36, 1 for active mercs)?
/*0041*/ int32 MercUnk02; // Unknown (normally see 1, but sometimes 2 or 0)
/*0045*/ int32 StanceCount; // Iterations of MercenaryStance_Struct - Normally 2 to 4 seen
/*0049*/ int32 MercUnk03; // Unknown (always 0 at merchant) - Seen on active merc: 93 a4 03 77, b8 ed 2f 26, 88 d5 8b c3, and 93 a4 ad 77
/*0053*/ int8 MercUnk04; // Seen 1
/*0054*/ MercenaryStance_Struct Stances[2]; // Count Varies, but hard set to 2 for now - From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
};
// Used by MercenaryDataUpdate_Struct
struct MercenaryData_Struct {
/*0000*/ int32 MercID; // ID unique to each type of mercenary (probably a DB id)
/*0004*/ int32 MercType; // From dbstr_us.txt - Apprentice (330000100), Journeyman (330000200), Master (330000300)
/*0008*/ int32 MercSubType; // From dbstr_us.txt - 330020105^23^Race: Guktan<br>Type: Healer<br>Confidence: High<br>Proficiency: Apprentice, Tier V...
/*0012*/ int32 PurchaseCost; // Purchase Cost (in gold)
/*0016*/ int32 UpkeepCost; // Upkeep Cost (in gold)
/*0020*/ int32 AltCurrencyCost; // Alternate Currency Purchase Cost? (all seen costs show N/A Bayle Mark) - Seen 0
/*0024*/ int32 AltCurrencyUpkeep; // Alternate Currency Upkeep Cost? (all seen costs show 1 Bayle Mark) - Seen 1
/*0028*/ int32 AltCurrencyType; // Alternate Currency Type? - 19^17^Bayle Mark^0 - Seen 19
/*0032*/ int8 MercUnk01; // Unknown (always see 0)
/*0033*/ sint32 TimeLeft; // Unknown (always see -1 at merchant) - Seen 900000 (15 minutes in ms for newly hired merc)
/*0037*/ int32 MerchantSlot; // Merchant Slot? Increments, but not always by 1 - May be for Merc Window Options (Seen 5, 36, 1 for active mercs)?
/*0041*/ int32 MercUnk02; // Unknown (normally see 1, but sometimes 2 or 0)
/*0045*/ int32 StanceCount; // Iterations of MercenaryStance_Struct - Normally 2 to 4 seen
/*0049*/ int32 MercUnk03; // Unknown (always 0 at merchant) - Seen on active merc: 93 a4 03 77, b8 ed 2f 26, 88 d5 8b c3, and 93 a4 ad 77
/*0053*/ int8 MercUnk04; // Seen 1
/*0054*/ MercenaryStance_Struct Stances[2]; // Count Varies, but hard set to 2 for now - From dbstr_us.txt - 1^24^Passive^0, 2^24^Balanced^0, etc (1 to 9 as of April 2012)
/*0000*/ int32 MercUnk05; // Seen 1 - Extra Merc Data field that differs from MercenaryListEntry_Struct
// MercUnk05 may be a field that is at the end of the packet only, even if multiple mercs are listed (haven't seen examples of multiple mercs owned at once)
};
My guess is that most of the smaller packets have not changed much if at all.
|
 |
|
 |

10-12-2012, 11:47 AM
|
Hill Giant
|
|
Join Date: Jul 2009
Location: Indianapolis
Posts: 228
|
|
Trev; will mercs piggyback off any of the bot code or are they standalone?
|

10-12-2012, 08:29 PM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
I will leave that type of thing up to the bot dev people like bad_captain. I assume they will get their own merc class that is basically just a copy of the bot class, but tweaked for mercs.
|
 |
|
 |

10-12-2012, 10:44 PM
|
Developer
|
|
Join Date: Feb 2009
Location: Cincinnati, OH
Posts: 512
|
|
I had a conversation about 2 years ago with Wildcard, who did a lot of the work on bots before me, and he had planned to change bots to be inherited off of mercs when they were finished. After doing a little bit of work on mercs, I'm not sure how easy or beneficial that would be, but I guess we'll see. The one real problem with bots (that won't be an issue with mercs), is the option to build without them, meaning a lot of code has to be rewritten or copied to get bots to work and still compile when they aren't enabled. Mercs should be built into the actual build so that they work a little more seamlessly than bots, even if they are less flexible.
On the other hand, with some changes on the live client, switching between multiple mercs, and editing the dbstring files, I think a lot could be done to merge the two. Incorporating the upkeep system into bots would be a benefit as well.
Once mercs get closer to being viable, I think the decision will have to be made whether to keep bots as is or inherit off of mercs, but unless someone can find other advantages to doing so, I see bots remaining as is.
|
 |
|
 |

10-12-2012, 10:53 PM
|
Developer
|
|
Join Date: Feb 2009
Location: Cincinnati, OH
Posts: 512
|
|
Note: your comment regarding the first field in the MercenaryMerchantRequest_Struct, is I believe the Merc ID, instead of RequestType.
|

10-13-2012, 06:58 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Quote:
Originally Posted by bad_captain
Note: your comment regarding the first field in the MercenaryMerchantRequest_Struct, is I believe the Merc ID, instead of RequestType.
|
Thanks, I corrected that one in the post.
|
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 02:07 PM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |