|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
Quests::Custom Custom Quests here |
 |
|
 |

07-01-2013, 08:40 PM
|
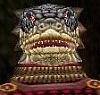 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
LUA Example #1 - Scaling an NPC
This is a module to scale an NPC, it goes in the lua_modules folder in the root directory of your EQEmu install. It should be called lua_scale.lua if you are testing out the example.
Code:
local scale_npc = {}
function scale_npc.scaleme(npc, level, zoneid, instanceid, scalerate, loottableid)
--Set up static constants, these are close to PEQ values:
local hpval = ((level * (11 + level)) * scalerate) / 100
local manaval = ((level * (11 + level)) * scalerate) / 100
local endurval = ((level * (11 + level)) * scalerate) / 100
local min_dmg = (((level + 2) + level) * scalerate) / 100
local max_dmg = (((level * 2) + level) * scalerate) / 100
local ac = ((28 + (4 * level)) * scalerate) / 100
local hp_regen_rate = (((level / 5) + 1) * scalerate) / 100
local mana_regen_rate = (((level / 5) + 1) * scalerate) / 100
local rawstats = ((75 + (level * 2)) * scalerate) / 100
--[[
Lua supports nice looking comments too, so why not tell people we are starting the actual scaling here?
Maybe include instance version at some point to the equation? :)
--]]
npc:AddLootTable(loottableid)
npc:ModifyNPCStat("str", tostring(rawstats))
npc:ModifyNPCStat("sta", tostring(rawstats))
npc:ModifyNPCStat("agi", tostring(rawstats))
npc:ModifyNPCStat("dex", tostring(rawstats))
npc:ModifyNPCStat("wis", tostring(rawstats))
npc:ModifyNPCStat("int", tostring(rawstats))
npc:ModifyNPCStat("cha", tostring(rawstats))
npc:ModifyNPCStat("ac", tostring(ac))
npc:ModifyNPCStat("max_hp", tostring(hpval))
npc:ModifyNPCStat("mana", tostring(manaval))
npc:ModifyNPCStat("min_hit", tostring(min_dmg))
npc:ModifyNPCStat("max_hit", tostring(max_dmg))
npc:ModifyNPCStat("hp_regen", tostring(hp_regen_rate))
npc:ModifyNPCStat("mana_regen", tostring(hp_regen_rate))
npc:ModifyNPCStat("level", tostring(level))
npc:ModifyNPCStat("healscale", tostring(healscale))
npc:ModifyNPCStat("spellscale", tostring(spellscale))
npc:Heal()
end
return scale_npc;
Note that LUA automatically converts string to int, but not int to string. You need to use conversion functions to do so.
Here is an example to scale an NPC by saying an integer to it.
Code:
function event_say(e)
e.self:Say("Scaleme!")
--This will scale an NPC to a level that you specify:
local luascale = require("lua_scale")
--Params: NPC to scale, Message, ZoneID, InstanceID, Scalerate, LootTable to assign
luascale.scaleme(e.self, e.message, eq.get_zone_id(), eq.get_zone_instance_id(), 100, 1)
end
More as I write them. PEQ has some good examples too, you should download their nightly repository here: https://drive.google.com/folderview?...p=sharing#list
|
 |
|
 |

07-02-2013, 11:31 AM
|
Discordant
|
|
Join Date: Dec 2005
Posts: 435
|
|
Thanks for that example.
|
 |
|
 |

07-03-2013, 08:10 PM
|
Administrator
|
|
Join Date: Sep 2006
Posts: 1,348
|
|
Just to add to the example you can take it and do something like:
Code:
function NPC:Scale(level, zoneid, instanceid, scalerate, loottableid)
--Set up static constants, these are close to PEQ values:
local hpval = ((level * (11 + level)) * scalerate) / 100
local manaval = ((level * (11 + level)) * scalerate) / 100
local endurval = ((level * (11 + level)) * scalerate) / 100
local min_dmg = (((level + 2) + level) * scalerate) / 100
local max_dmg = (((level * 2) + level) * scalerate) / 100
local ac = ((28 + (4 * level)) * scalerate) / 100
local hp_regen_rate = (((level / 5) + 1) * scalerate) / 100
local mana_regen_rate = (((level / 5) + 1) * scalerate) / 100
local rawstats = ((75 + (level * 2)) * scalerate) / 100
--[[
Lua supports nice looking comments too, so why not tell people we are starting the actual scaling here?
Maybe include instance version at some point to the equation? :)
--]]
self:AddLootTable(loottableid)
self:ModifyNPCStat("str", tostring(rawstats))
self:ModifyNPCStat("sta", tostring(rawstats))
self:ModifyNPCStat("agi", tostring(rawstats))
self:ModifyNPCStat("dex", tostring(rawstats))
self:ModifyNPCStat("wis", tostring(rawstats))
self:ModifyNPCStat("int", tostring(rawstats))
self:ModifyNPCStat("cha", tostring(rawstats))
self:ModifyNPCStat("ac", tostring(ac))
self:ModifyNPCStat("max_hp", tostring(hpval))
self:ModifyNPCStat("mana", tostring(manaval))
self:ModifyNPCStat("min_hit", tostring(min_dmg))
self:ModifyNPCStat("max_hit", tostring(max_dmg))
self:ModifyNPCStat("hp_regen", tostring(hp_regen_rate))
self:ModifyNPCStat("mana_regen", tostring(hp_regen_rate))
self:ModifyNPCStat("level", tostring(level))
self:ModifyNPCStat("healscale", tostring(healscale))
self:ModifyNPCStat("spellscale", tostring(spellscale))
self:Heal()
end
and in your global/script_init.lua add
require("lua_scale")
Then you could literally do
Code:
function event_say(e)
e.self:Say("Scaleme!")
e.self:Scale(e.message, eq.get_zone_id(), eq.get_zone_instance_id(), 100, 1)
end
Inheritance issues can sometimes make it a bit tricky though as these wont be in the metatable lookup that deals with inheritance.
|
 |
|
 |
 |
|
 |

07-03-2013, 11:52 PM
|
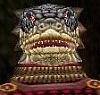 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Quote:
Originally Posted by KLS
Just to add to the example you can take it and do something like:
Code:
function NPC:Scale(level, zoneid, instanceid, scalerate, loottableid)
--Set up static constants, these are close to PEQ values:
local hpval = ((level * (11 + level)) * scalerate) / 100
local manaval = ((level * (11 + level)) * scalerate) / 100
local endurval = ((level * (11 + level)) * scalerate) / 100
local min_dmg = (((level + 2) + level) * scalerate) / 100
local max_dmg = (((level * 2) + level) * scalerate) / 100
local ac = ((28 + (4 * level)) * scalerate) / 100
local hp_regen_rate = (((level / 5) + 1) * scalerate) / 100
local mana_regen_rate = (((level / 5) + 1) * scalerate) / 100
local rawstats = ((75 + (level * 2)) * scalerate) / 100
--[[
Lua supports nice looking comments too, so why not tell people we are starting the actual scaling here?
Maybe include instance version at some point to the equation? :)
--]]
self:AddLootTable(loottableid)
self:ModifyNPCStat("str", tostring(rawstats))
self:ModifyNPCStat("sta", tostring(rawstats))
self:ModifyNPCStat("agi", tostring(rawstats))
self:ModifyNPCStat("dex", tostring(rawstats))
self:ModifyNPCStat("wis", tostring(rawstats))
self:ModifyNPCStat("int", tostring(rawstats))
self:ModifyNPCStat("cha", tostring(rawstats))
self:ModifyNPCStat("ac", tostring(ac))
self:ModifyNPCStat("max_hp", tostring(hpval))
self:ModifyNPCStat("mana", tostring(manaval))
self:ModifyNPCStat("min_hit", tostring(min_dmg))
self:ModifyNPCStat("max_hit", tostring(max_dmg))
self:ModifyNPCStat("hp_regen", tostring(hp_regen_rate))
self:ModifyNPCStat("mana_regen", tostring(hp_regen_rate))
self:ModifyNPCStat("level", tostring(level))
self:ModifyNPCStat("healscale", tostring(healscale))
self:ModifyNPCStat("spellscale", tostring(spellscale))
self:Heal()
end
and in your global/script_init.lua add
require("lua_scale")
Then you could literally do
Code:
function event_say(e)
e.self:Say("Scaleme!")
e.self:Scale(e.message, eq.get_zone_id(), eq.get_zone_instance_id(), 100, 1)
end
Inheritance issues can sometimes make it a bit tricky though as these wont be in the metatable lookup that deals with inheritance.
|
Interesting addition, KLS.
Hopefully this will cut down the need for so many functions that we generally export to perl - except they'll be faster.
|
 |
|
 |
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 12:31 PM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |