|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
 |
|
 |

10-30-2010, 06:57 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
COMMITTED: Expendable, Quest, Racial/Bloodline & Shroud AAs
So Ive been spending some off time updating the AA Database so we can have all the AAs as they were intended up to SoD/Underfoot but its going to be a huge update so thought I would split this off first.
Here's whats been updated:
1.) Expendable AAs - Set the special_category in the altadv_vars table to 7 and the AA will show as expendable as well as un-purchase itself when you activate it(like live). Also utilizes the extended profile(thx secrets) to keep the expended aas counted in the spent AA total.
2.) Quest AAs - Like Trials of Mata Muram AA. Set the special_category to 1(Quest Only) or 2(Progression). These AA will remain hidden from view until you have been granted 1 level in them(using $client->AssignAA, see below). They also remain greyed out and are unpurchasable(they show a cost of -1 to make it clear).
3.) Racial/Bloodline AAs - Mainly done for the Drakkin breath weapons however I included support for racial AAs(not like live but why give drakkins all the fun). Set the special_category to 8 and then the aa_expansion entry is used as the race/bloodline. Races are entered as the race, bloodlines are entered + 523(drakkin base race + 1).
eg.
Code:
Human = 1
Barbarian = 2
Erud = 3
Wood = 4
High = 5
Dark = 6
Half = 7
Dwarf = 8
Troll = 9
Ogre = 10
Halfing = 11
Gnome = 12
Iksar = 128
Vahshir = 130
Frog = 330
Drakkin = 522
// Bloodlines
DrakkinRed(0) = 523
DrakkinBlack(1) = 524
DrakkinBlue(2) = 525
DrakkinGreen(3) = 526
DrakkinWhite(4) = 527
DrakkinGold(5) = 528
4.) New object... Client::AssignAA(AA_ID, Value)
This command will grant or remove a specific AA.
Example of use... set an AA to quest or progression(1 or 2). Create script with $client->AssignAA(AA_ID, 1); where AA_ID is the skill_id from the altadv_vars table. The AA will not show on the list prior to executing the script and after it will be visible.
5.) Support for 50 levels of passive AA and 20 level of activated AAs. This is a base for the coming AA Revamp whereby all the AA are consolidated into 1 AA(ie no 4x Combat Stability, instead -> Combat Stability with 33 ranks) like live.
6.) Very beginning of Shroud AAs. Special category 3(Passive) and 4(activated) are for shroud AAs, I included a check to block them from showing for players. In the future, should be possible to display the AA list based on whether the player is playing as themselves or a shroud.
NOTE: The default AA DB doesnt contain any Expendable, Progression, Racial or Shroud AAs. Within a week or so I should have them updated and will post that as well, complete up to SoD(some Underfoot as well, just wont be visible as they show as unknown). Maybe 2 weeks. Going to be removing as much hard coding as possible as well which will take some bonus re-writing so could take a while.
SQL(need to alter aa_expansion slightly so it can hold numbers past 255):
Code:
ALTER TABLE `altadv_vars` CHANGE COLUMN `aa_expansion` `aa_expansion` SMALLINT(3) UNSIGNED NOT NULL DEFAULT '3' AFTER `cost_inc`;
CODE:
Code:
Index: common/extprofile.h
===================================================================
--- common/extprofile.h (revision 1709)
+++ common/extprofile.h (working copy)
@@ -46,6 +46,7 @@
uint32 aa_effects;
uint32 perAA; //% of exp going to AAs
+ uint32 expended_aa; // Total of expended AA
};
#pragma pack()
Index: zone/AA.cpp
===================================================================
--- zone/AA.cpp (revision 1709)
+++ zone/AA.cpp (working copy)
@@ -96,7 +96,7 @@
if(!aa2)
{
- for(int i = 1;i < 5; ++i)
+ for(int i = 1;i < MAX_AA_ACTION_RANKS; ++i)
{
int a = activate - i;
@@ -158,7 +158,7 @@
if(!aa2){
int i;
int a;
- for(i=1;i<5;i++){
+ for(i=1;i<MAX_AA_ACTION_RANKS;i++){
a = activate - i;
if(a <= 0)
break;
@@ -295,6 +295,19 @@
return;
}
}
+
+ // Check if AA is expendable
+ if (aas_send[activate]->special_category == 7)
+ {
+ // Add the AA cost to the extended profile to track overall total
+ m_epp.expended_aa += aas_send[activate]->cost;
+ SetAA(activate, 0);
+
+ Save();
+
+ SendAA(activate);
+ SendAATable();
+ }
}
void Client::HandleAAAction(aaID activate) {
@@ -886,7 +899,7 @@
//hunt for a lower level...
int i;
int a;
- for(i=1;i<15;i++){
+ for(i=1;i<MAX_AA_ACTION_RANKS;i++){
a = action->ability - i;
if(a <= 0)
break;
@@ -898,6 +911,9 @@
}
if(aa2 == NULL)
return; //invalid ability...
+
+ if(aa2->special_category == 1 || aa2->special_category == 2)
+ return; // Not purchasable progression style AAs
int32 cur_level = GetAA(aa2->id);
if((aa2->id + cur_level) != action->ability) { //got invalid AA
@@ -1036,7 +1052,35 @@
if(!(classes & (1 << GetClass())) && (GetClass()!=BERSERKER || saa2->berserker==0)){
return;
}
+
+ // Beginning of Shroud AAs, these categories are for Passive and Active Shroud AAs
+ // Eventually with a toggle we could have it show player list or shroud list
+ if (saa2->special_category == 3 || saa2->special_category == 4)
+ return;
+ // Check for racial/Drakkin blood line AAs
+ if (saa2->special_category == 8)
+ {
+ int32 client_race = this->GetBaseRace();
+
+ // Drakkin Bloodlines
+ if (saa2->aa_expansion > 522)
+ {
+ if (client_race != 522)
+ return; // Check for Drakkin Race
+
+ int heritage = this->GetDrakkinHeritage() + 523; // 523 = Drakkin Race(522) + Bloodline
+
+ if (heritage != saa2->aa_expansion)
+ return;
+ }
+ // Racial AAs
+ else if (client_race != saa2->aa_expansion)
+ {
+ return;
+ }
+ }
+
int size=sizeof(SendAA_Struct)+sizeof(AA_Ability)*saa2->total_abilities;
uchar* buffer = new uchar[size];
SendAA_Struct* saa=(SendAA_Struct*)buffer;
@@ -1046,11 +1090,21 @@
saa->spellid=0xFFFFFFFF;
value=GetAA(saa->id);
+
+ int quest_aa = 0;
+ // Make quest & progression AA not visible until granted 1 level.
+ if (saa2->special_category == 1 || saa2->special_category == 2 )
+ {
+ if (value == 0)
+ return;
+ quest_aa = 1;
+ }
+
int32 orig_val = value;
bool dump = false;
if(value){
dump = true;
- if(value < saa->max_level){
+ if(value < saa->max_level && !quest_aa){
saa->id+=value;
saa->next_id=saa->id+1;
value++;
@@ -1066,6 +1120,11 @@
for(int i=0;i<value;i++){
saa->cost2 += saa2->cost + (saa2->cost_inc * i);
}
+ if (quest_aa)
+ {
+ saa->cost = 0xFFFFFFFF;
+ saa->cost2 = 0xFFFFFFFF;
+ }
}
database.FillAAEffects(saa);
Index: zone/client.cpp
===================================================================
--- zone/client.cpp (revision 1709)
+++ zone/client.cpp (working copy)
@@ -493,10 +493,10 @@
int spentpoints=0;
for(int a=0;a < MAX_PP_AA_ARRAY;a++) {
int32 points = aa[a]->value;
- if(points > 20) //sanity check if you want an aa to have over 20 ranks you'll need to up this.
+ if(points > HIGHEST_AA_VALUE) // Unifying this
{
- aa[a]->value = 20;
- points = 20;
+ aa[a]->value = HIGHEST_AA_VALUE;
+ points = HIGHEST_AA_VALUE;
}
if (points > 0) {
SendAA_Struct* curAA = zone->FindAA(aa[a]->AA-aa[a]->value+1);
@@ -506,7 +506,7 @@
}
}
}
- m_pp.aapoints_spent = spentpoints;
+ m_pp.aapoints_spent = spentpoints + m_epp.expended_aa;
if (GetHP() <= 0) {
m_pp.cur_hp = GetMaxHP();
Index: zone/features.h
===================================================================
--- zone/features.h (revision 1709)
+++ zone/features.h (working copy)
@@ -245,7 +245,7 @@
//level is the only valid variable to use
#define EXP_FORMULA level*level*75*35/10
-#define HIGHEST_AA_VALUE 11
+#define HIGHEST_AA_VALUE 50
//Leadership AA experience points
#define GROUP_EXP_PER_POINT 1000
Index: zone/perl_client.cpp
===================================================================
--- zone/perl_client.cpp (revision 1709)
+++ zone/perl_client.cpp (working copy)
@@ -4669,6 +4669,34 @@
XSRETURN_EMPTY;
}
+XS(XS_Client_AssignAA); /* prototype to pass -Wmissing-prototypes */
+XS(XS_Client_AssignAA)
+{
+ dXSARGS;
+ if (items < 2 || items > 3)
+ Perl_croak(aTHX_ "Usage: Client::AssignAA(THIS, aaskillid, value)");
+ {
+ Client * THIS;
+ int32 aaskillid = SvUV(ST(1));
+ int32 value = SvUV(ST(2));
+
+ if (sv_derived_from(ST(0), "Client")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(Client *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type Client");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ THIS->SetAA(aaskillid, value);
+ THIS->Save();
+ THIS->SendAA(aaskillid);
+ THIS->SendAATable();
+ }
+ XSRETURN_EMPTY;
+}
+
#ifdef __cplusplus
extern "C"
#endif
@@ -4860,6 +4888,7 @@
newXSproto(strcpy(buf, "NPCSpawn"), XS_Client_NPCSpawn, file, "$$$;$");
newXSproto(strcpy(buf, "GetIP"), XS_Client_GetIP, file, "$");
newXSproto(strcpy(buf, "AddLevelBasedExp"), XS_Client_AddLevelBasedExp, file, "$$;$");
+ newXSproto(strcpy(buf, "AssignAA"), XS_Client_AssignAA, file, "$$$");
XSRETURN_YES;
}
|
 |
|
 |
 |
|
 |

10-30-2010, 11:57 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
Always find an error after the edit timer is up... I changed the function to
Client::IncrementAA(AA_ID)
The removing of AA levels is kind of funky so I just removed that ability and simplified it so that you only need to put in the Skill_ID from altadv_vars. Also I forgot to make sure that bonuses were calculated again.
New Code:
Code:
Index: common/extprofile.h
===================================================================
--- common/extprofile.h (revision 1709)
+++ common/extprofile.h (working copy)
@@ -46,6 +46,7 @@
uint32 aa_effects;
uint32 perAA; //% of exp going to AAs
+ uint32 expended_aa; // Total of expended AA
};
#pragma pack()
Index: zone/AA.cpp
===================================================================
--- zone/AA.cpp (revision 1709)
+++ zone/AA.cpp (working copy)
@@ -96,7 +96,7 @@
if(!aa2)
{
- for(int i = 1;i < 5; ++i)
+ for(int i = 1;i < MAX_AA_ACTION_RANKS; ++i)
{
int a = activate - i;
@@ -158,7 +158,7 @@
if(!aa2){
int i;
int a;
- for(i=1;i<5;i++){
+ for(i=1;i<MAX_AA_ACTION_RANKS;i++){
a = activate - i;
if(a <= 0)
break;
@@ -295,6 +295,19 @@
return;
}
}
+
+ // Check if AA is expendable
+ if (aas_send[activate]->special_category == 7)
+ {
+ // Add the AA cost to the extended profile to track overall total
+ m_epp.expended_aa += aas_send[activate]->cost;
+ SetAA(activate, 0);
+
+ Save();
+
+ SendAA(activate);
+ SendAATable();
+ }
}
void Client::HandleAAAction(aaID activate) {
@@ -886,7 +899,7 @@
//hunt for a lower level...
int i;
int a;
- for(i=1;i<15;i++){
+ for(i=1;i<MAX_AA_ACTION_RANKS;i++){
a = action->ability - i;
if(a <= 0)
break;
@@ -898,6 +911,9 @@
}
if(aa2 == NULL)
return; //invalid ability...
+
+ if(aa2->special_category == 1 || aa2->special_category == 2)
+ return; // Not purchasable progression style AAs
int32 cur_level = GetAA(aa2->id);
if((aa2->id + cur_level) != action->ability) { //got invalid AA
@@ -1036,7 +1052,35 @@
if(!(classes & (1 << GetClass())) && (GetClass()!=BERSERKER || saa2->berserker==0)){
return;
}
+
+ // Beginning of Shroud AAs, these categories are for Passive and Active Shroud AAs
+ // Eventually with a toggle we could have it show player list or shroud list
+ if (saa2->special_category == 3 || saa2->special_category == 4)
+ return;
+ // Check for racial/Drakkin blood line AAs
+ if (saa2->special_category == 8)
+ {
+ int32 client_race = this->GetBaseRace();
+
+ // Drakkin Bloodlines
+ if (saa2->aa_expansion > 522)
+ {
+ if (client_race != 522)
+ return; // Check for Drakkin Race
+
+ int heritage = this->GetDrakkinHeritage() + 523; // 523 = Drakkin Race(522) + Bloodline
+
+ if (heritage != saa2->aa_expansion)
+ return;
+ }
+ // Racial AAs
+ else if (client_race != saa2->aa_expansion)
+ {
+ return;
+ }
+ }
+
int size=sizeof(SendAA_Struct)+sizeof(AA_Ability)*saa2->total_abilities;
uchar* buffer = new uchar[size];
SendAA_Struct* saa=(SendAA_Struct*)buffer;
@@ -1046,6 +1090,13 @@
saa->spellid=0xFFFFFFFF;
value=GetAA(saa->id);
+
+ // Hide Quest/Progression AAs unless player has been granted the first level.
+ if ((saa2->special_category == 1 || saa2->special_category == 2 ) && (value == 0))
+ {
+ return;
+ }
+
int32 orig_val = value;
bool dump = false;
if(value){
Index: zone/client.cpp
===================================================================
--- zone/client.cpp (revision 1709)
+++ zone/client.cpp (working copy)
@@ -493,10 +493,10 @@
int spentpoints=0;
for(int a=0;a < MAX_PP_AA_ARRAY;a++) {
int32 points = aa[a]->value;
- if(points > 20) //sanity check if you want an aa to have over 20 ranks you'll need to up this.
+ if(points > HIGHEST_AA_VALUE) // Unifying this
{
- aa[a]->value = 20;
- points = 20;
+ aa[a]->value = HIGHEST_AA_VALUE;
+ points = HIGHEST_AA_VALUE;
}
if (points > 0) {
SendAA_Struct* curAA = zone->FindAA(aa[a]->AA-aa[a]->value+1);
@@ -506,7 +506,7 @@
}
}
}
- m_pp.aapoints_spent = spentpoints;
+ m_pp.aapoints_spent = spentpoints + m_epp.expended_aa;
if (GetHP() <= 0) {
m_pp.cur_hp = GetMaxHP();
Index: zone/features.h
===================================================================
--- zone/features.h (revision 1709)
+++ zone/features.h (working copy)
@@ -245,7 +245,7 @@
//level is the only valid variable to use
#define EXP_FORMULA level*level*75*35/10
-#define HIGHEST_AA_VALUE 11
+#define HIGHEST_AA_VALUE 50
//Leadership AA experience points
#define GROUP_EXP_PER_POINT 1000
Index: zone/perl_client.cpp
===================================================================
--- zone/perl_client.cpp (revision 1709)
+++ zone/perl_client.cpp (working copy)
@@ -4669,6 +4669,45 @@
XSRETURN_EMPTY;
}
+XS(XS_Client_IncrementAA);
+XS(XS_Client_IncrementAA)
+{
+ dXSARGS;
+ if (items < 2 || items > 2)
+ Perl_croak(aTHX_ "Usage: Client::IncrementAA(THIS, aaskillid)");
+ {
+ Client * THIS;
+ int32 aaskillid = SvUV(ST(1));
+
+ if (sv_derived_from(ST(0), "Client")) {
+ IV tmp = SvIV((SV*)SvRV(ST(0)));
+ THIS = INT2PTR(Client *,tmp);
+ }
+ else
+ Perl_croak(aTHX_ "THIS is not of type Client");
+ if(THIS == NULL)
+ Perl_croak(aTHX_ "THIS is NULL, avoiding crash.");
+
+ SendAA_Struct* aa2 = zone->FindAA(aaskillid);
+
+ if(aa2 == NULL)
+ Perl_croak(aTHX_ "Invalid AA.");
+
+ if(THIS->GetAA(aaskillid) == aa2->max_level)
+ Perl_croak(aTHX_ "AA at Max already.");
+
+ THIS->SetAA(aaskillid, THIS->GetAA(aaskillid)+1);
+
+ THIS->Save();
+
+ THIS->SendAA(aaskillid);
+ THIS->SendAATable();
+ THIS->SendAAStats();
+ THIS->CalcBonuses();
+ }
+ XSRETURN_EMPTY;
+}
+
#ifdef __cplusplus
extern "C"
#endif
@@ -4860,6 +4899,7 @@
newXSproto(strcpy(buf, "NPCSpawn"), XS_Client_NPCSpawn, file, "$$$;$");
newXSproto(strcpy(buf, "GetIP"), XS_Client_GetIP, file, "$");
newXSproto(strcpy(buf, "AddLevelBasedExp"), XS_Client_AddLevelBasedExp, file, "$$;$");
+ newXSproto(strcpy(buf, "IncrementAA"), XS_Client_IncrementAA, file, "$$");
XSRETURN_YES;
}
|
 |
|
 |
 |
|
 |

11-12-2010, 03:44 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
Just wanted to post alittle information on how this all works now that it has been committed.
Expendable AAs - Any AA set to special_category 7 is expendable so once its activated it gets reset. You can make multiple level AAs(Live only has single level but I coded it to support multiple levels) however whenever its activated they will lose all levels in it.
Quest/Progression AAs - these can be 2 kinds, like DoN Progression where there are 5 AAs and at the end you have 5 AAs or like Demiplane Progression where there are 5 AAs but you only ever have one visible at a time.
For DoN Style, set special_category to 1/2 and then use a script like:
Code:
sub EVENT_xxxx
{
$client->IncrementAA(1361);
}
If you look in DB you will see that 1361 is Gift of the Dark Reign. This will make the AA visible to the client and grant a point in it.
For DoDh style progression, you need to set the special_category to 1/2 as well as set the aa_expansion field to be the same for all AAs you want to be grouped, in the DB all DoDh AAs are set to 5 so only 1 quest/progression AA will be visible depending on what is the highest rank obtained by the player. To do this, use the same script as above and when you increment the next AA in the line it will automatically hide the previous AA(slight bug, the previous AA wont disappear from the window until the client camps).
Racial/Bloodline AAs - use special_category 8 and then input the race into the aa_expansion field. These AAs are invisible to everybody except those races and by default they are unpurchasable, if you want them to be purchasable you need to set a cost(0 cost = not buyable). You use the same IncrementAA function to increase their levels.
For drakkin breath AAs, you will need to use a script like:
Code:
sub EVENT_xxx
{
if($client->GetBaseRace() == 522) {
my $bloodline = $client->GetDrakkinHeritage();
my $aa_to_use = 0;
if($bloodline == 0)
$aa_to_use = 5150; ## This is the first breath weapon
elsif($bloodline == 1)
$aa_to_use = 5165; ## This is the second breath weapon
etc..
if($aa_to_use > 0)
$client->IncrementAA($aa_to_use);
}
}
Also can use the GetAALevel to restrict or whatever. Like if you wanted to have the zonewide AEs in demiplane landing based on their progression AAs, you could use that function to determine which AE should land on said player.
|
 |
|
 |

11-12-2010, 05:10 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
forgot to include an update to the altadv_vars table in the 1720 update so the drakkin breath weapons werent set correctly, make sure to grab the sql from 1721 to fix the issue.
|

11-14-2010, 05:53 PM
|
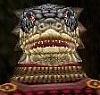 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
DoT critical chance actually needs to be a negative number turned positive... this is never done in TryDoTCritical, and the only spell that I can see using it (I only skimmed over the spellfile) was rising terror. It's a negative number there.
Also, I had to revert the melee critical chance stuff in my local copy otherwise melee were critting every hit if they had a critical.
For some reason heals were doing nothing, had to revert the healrate stuff as well in my local copy.
Other than that looks good. Haven't looked at the rest. Definitely wouldn't try it on a server until the issues get worked out though.
|

11-14-2010, 08:05 PM
|
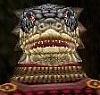 |
Demi-God
|
|
Join Date: May 2007
Location: b
Posts: 1,450
|
|
Also noticing 100% crit chance on melee with Underfoot items ingame.
|

11-14-2010, 09:08 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
Pretty sure DoT crit chance is positive, I checked lucy but the only spell named rising terror doesnt have that effect in it(I checked all 5 copies even!). A well known spell that increases dot crit chance is Intensity of the Resolute and in that spell its positive.
Before I work on any effect I always run a query that searches all effectids for the specifc effect so I make sure I implement it with the actual spells in mind. Obviously its not a perfect system(not sure whats going on with melee/heals off the top of my head, most likely faulty logic though).
Unfortunately I am away this week so dont have much time to work on code, however if you find the issue before then I'm sure trev will commit it. Otherwise Ill see what I can do when Im back. Safe to say there will be issues caused by so many updates so quickly, so more testing is probably in order.
|
 |
|
 |

11-15-2010, 06:46 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
I think I see the issue (or at least an issue) with melee criticals.
The problem appears to be due to the changes in the diff here (starting at line 3832):
http://code.google.com/p/projecteqem...one/attack.cpp
It looks like at least one problem is that the rules for crits are all done as floats and defaults can be seen here:
http://code.google.com/p/projecteqem...on/ruletypes.h
With the new changes, I think they either need to multiply the rule values by 100 (either the variables in the code or an SQL update to do it, as well as defaults in ruletypes.h). Otherwise, the defaults and rules become very minor differences as apposed to what they should be (and were before) by a factor of 100. Also, with changes from using decimals and floats to using ints and whole numbers there, I think the divider of 100 needs to be changed to 1000. As I read it, it looks like crits may be getting a 100X multiplier right now. I will have to re-read it to make sure I understand exactly what is going on before making any changes, though.
I think making the needed corrections there should at least resolve one of the issues with the recent updates. Considering the number of changes, a few issues are to be expected (IMO anyway). Knocking out the fixes shouldn't be too bad and the plus side of these changes way outweighs the issues at this point. These are some much needed changes and it is awesome to see someone interested in doing them (thanks, Caryatis!)
As far as issues with the recent updates go, I haven't noticed anything that would require an immediate revert due to game-breaking issues, but that may depend on the server running them and their setup.
Here are some of the reported issues so far on my server that might help keep track of what needs to be looked at:
http://stormhavenserver.com/forums/viewtopic.php?t=1870
I may need to start a new thread to discuss issues with the latest changes, as they aren't all really directed at this one submission. Since Caryatis is out on vacation this week, I am going to see how many issues I can find and resolve and if anyone else wants to help, feel free. I think Caryatis will be more than willing to work on any remaining issues when he gets back.
Last edited by trevius; 11-15-2010 at 08:26 AM..
|
 |
|
 |

11-15-2010, 06:56 AM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
Also, I am not sure about the changes here:
http://code.google.com/p/projecteqem...ne/bonuses.cpp
At lines 1051 and 1132 (from the left side), it looks like instead of using the highest spell effect mod, it adds all of them up. I am not sure if that is how it is supposed to work, but seems to me it would only use the highest value, or at least cap it somehow.
|
 |
|
 |

11-20-2010, 08:43 PM
|
Dragon
|
|
Join Date: May 2009
Location: Milky Way
Posts: 541
|
|
The critical hit issue has been resolved. The problem was the fact that CriticalHitChance was being stored in a single variable while the spells that utilize this effect come with skill specific tags(also with the change to way the effect was added, it was adding all of the bonuses together).
For example Cleave:
Quote:
1: Increase Chance to Critical Hit by 40% with 1H Blunt
2: Increase Chance to Critical Hit by 40% with 1H Slashing
3: Increase Chance to Critical Hit by 40% with 2H Blunt
4: Increase Chance to Critical Hit by 40% with 2H Slashing
5: Increase Chance to Critical Hit by 40% with Backstab
6: Increase Chance to Critical Hit by 40% with Bash
7: Increase Chance to Critical Hit by 40% with Flying Kick
8: Increase Chance to Critical Hit by 40% with Hand To Hand
9: Increase Chance to Critical Hit by 40% with Kick
10: Increase Chance to Critical Hit by 40% with Piercing
11: Increase Chance to Critical Hit by 40% with 2H Piercing
|
I made 2 changes to fix this:
1.) Stored the data in an array like the other effects Ive added(skilldmgtaken) which allows for the bonuses to match the skills.
2.) Added a check so that item bonuses will not stack with themselves(ie Cleave I and Cleave II won't stack) but spell bonuses will stack(so things like speed of ellowind(1% bonus to crit) will stack with other crit bonus spells).
Also tidied up all the variables involved so that we have ints across the board(doesnt seem like we need floats for this).
Edit: Healrate fix went in as well, all issues I can find have been resolved so if you find anymore please let me know.
|
 |
|
 |
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 01:17 PM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |