|
|
 |
 |
 |
 |
|
 |
 |
|
 |
 |
|
 |
|
Quests::Q&A This is the quest support section |
 |
|
 |

01-09-2010, 05:01 AM
|
Fire Beetle
|
|
Join Date: Nov 2009
Posts: 21
|
|
Race dependant teleport
So what I am trying to do is create a teleporter that will teleport people to their starting cities according to race. So far it appears as though the command i need is "if($race eq 'Dark Elf')" so I'm assuming the code should look as follows:
Code:
sub EVENT_SAY
{
if ($text =~/Hail/i)
{
quest::say ("Good day to you, $name. Do you want to [go] home?");
}
if ($text =~/go/i)
if ($race eq 'Dark Elf')
{
quest::MovePC(202, -118,-193,-156)
}
}
now im assuming this would work for a single race, however how would i set it up where it does a race check, determines race, and sets it to quest::movepc(zoneid, X, Y, Z) i feel like there should be someway to declare a THEN statement but im not sure how in perl. The reason i want this is i am using a central hub where people can get spells/train/epic quests/armor quests/ (think EZ server) while still requiring people to play through the original game.
P.S. sorry if i offended the gods with my ugly perl format, ideas and criticism encouraged!
|
 |
|
 |

01-09-2010, 08:03 AM
|
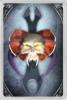 |
Developer
|
|
Join Date: Mar 2003
Posts: 1,498
|
|
Code:
if (condition) {
then this;
}
elsif (this other) {
this instead;
}
else {
finally this;
}
Also use movepc() instead of MovePC()
|
 |
|
 |

01-09-2010, 08:34 AM
|
Fire Beetle
|
|
Join Date: Nov 2009
Posts: 21
|
|
Code:
sub EVENT_SAY
{
if ($text =~/Hail/i)
{
quest::say ("Good day to you, $name. Do you want to [go] home?");
}
if ($text =~/go/i) && ($race eq 'Dark Elf') {
quest::movepc(40, 156.92, -2.94, 31.75) ;
}
elsif ($text =~/go/i) && ($race eq 'Troll') {
quest::movepc(52, 0, -100, 4) ;
}
elsif ($text =~/go/i) && ($race eq 'Wood Elf') {
quest::movepc(54, 10, -20, 0) ;
}
elsif ($text =~/go/i) && ($race eq 'Half-Elf') {
quest::movepc(54, 10, -20, 0) ;
}
elsif ($text =~/go/i) && ($race eq 'Vah Shir') {
quest::movepc(54, 10, -20, 0) ;
}
elsif ($text =~/go/i) && ($race eq 'Froglok') {
quest::movepc(105, -18, -123, -16) ;
}
elsif ($text =~/go/i) && ($race eq 'High Elf') {
quest::movepc(61, 94, -25, 3.75) ;
}
elsif ($text =~/go/i) && ($race eq 'Gnome') {
quest::movepc(55, -35, -47, 4) ;
}
elsif ($text =~/go/i) && ($race eq 'Human') {
quest::movepc(8, 211, -296, 4) ;
}
elsif ($text =~/go/i) && ($race eq 'Halfling') {
quest::movepc(19, 45.3, 1.6, 3.8) ;
}
elsif ($text =~/go/i) && ($race eq 'Erudite') {
quest::movepc(24, -309.75, 109.64, 23.75) ;
}
elsif ($text =~/go/i) && ($race eq 'Drakkin') {
quest::movepc(394, -550, -430, 80) ;
}
elsif ($text =~/go/i) && ($race eq 'Iksar') {
quest::movepc(106, -416, 1343, 4) ;
}
elsif ($text =~/go/i) && ($race eq 'Troll') {
quest::movepc(52, 0, -100, 4) ;
}
elsif ($text =~/go/i) && ($race eq 'Ogre') {
quest::movepc(37, -99, -345, 4) ;
}
elsif ($text =~/go/i) && ($race eq 'Barbarian') {
quest::movepc(29, 0, 0, 3.75) ;
}
else ($text =~/go/i) && ($race eq 'Dwarf') {
quest::movepc(60, -2, -18, 3.75) ;
}
}
like this? This is my general idea of the perl i want to work. yes i realize vah shir and Half-Elf are stuck together with wood elves.
|
 |
|
 |

01-09-2010, 10:32 AM
|
 |
Discordant
|
|
Join Date: Mar 2009
Location: Ottawa
Posts: 495
|
|
Your last else() should be an elsif() like the others. You can't have else(condition).
Also, perl might require you to use double quotes around $race eq 'something', I'm not sure. In most languages that I know, single quotes denotes a single character, double quotes a string.
|
 |
|
 |

01-09-2010, 11:29 AM
|
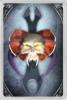 |
Developer
|
|
Join Date: Mar 2003
Posts: 1,498
|
|
Really, you don't need any esle type statements unless you are wanting to just stop the process. There shouldn't be any characters that have 2 classes.
Code:
sub EVENT_SAY
{
if ($text =~/Hail/i)
{
quest::say ("Good day to you, $name. Do you want to [go] home?");
}
if ($text =~/go/i) {
if ($race eq 'Dark Elf') {
quest::movepc(40, 156.92, -2.94, 31.75) ;
}
if ($race eq 'Troll') {
quest::movepc(52, 0, -100, 4) ;
}
if ($race eq 'Wood Elf') {
quest::movepc(54, 10, -20, 0) ;
}
if ($race eq 'Half-Elf') {
quest::movepc(54, 10, -20, 0) ;
}
if ($race eq 'Vah Shir') {
quest::movepc(54, 10, -20, 0) ;
}
if ($race eq 'Froglok') {
quest::movepc(105, -18, -123, -16) ;
}
if ($race eq 'High Elf') {
quest::movepc(61, 94, -25, 3.75) ;
}
if ($race eq 'Gnome') {
quest::movepc(55, -35, -47, 4) ;
}
if ($race eq 'Human') {
quest::movepc(8, 211, -296, 4) ;
}
if ($race eq 'Halfling') {
quest::movepc(19, 45.3, 1.6, 3.8) ;
}
if ($race eq 'Erudite') {
quest::movepc(24, -309.75, 109.64, 23.75) ;
}
if ($race eq 'Drakkin') {
quest::movepc(394, -550, -430, 80) ;
}
if ($race eq 'Iksar') {
quest::movepc(106, -416, 1343, 4) ;
}
if ($race eq 'Troll') {
quest::movepc(52, 0, -100, 4) ;
}
if ($race eq 'Ogre') {
quest::movepc(37, -99, -345, 4) ;
}
if ($race eq 'Barbarian') {
quest::movepc(29, 0, 0, 3.75) ;
}
if ($race eq 'Dwarf') {
quest::movepc(60, -2, -18, 3.75) ;
}
}
}
|
 |
|
 |
 |
|
 |

01-11-2010, 01:41 PM
|
Fire Beetle
|
|
Join Date: Nov 2009
Posts: 21
|
|
the above script doesn't work, neither does inserting the ID numbers for the race as well, this is what i have put in, i also had to make a couple corrections.
Code:
#Perl Script for Albert to teleport People to their respective starting cities
sub EVENT_SAY {
if ($text =~/Hail/i)
{
quest::say ("Good day to you, $name. I imagine you have talked to Death by now? If you haven't, you really should seek him out. If you have, are you ready to [go home]?");
}
if ($text =~/go home/i) {
if ($race eq '6')
{
quest::MovePC(40, 156.92, -2.94, 31.75) ;
}
if ($race eq '9')
{
quest::MovePC(52, 0, -100, 4) ;
}
if ($race eq '4')
{
quest::MovePC(54, 10, -20, 0) ;
}
if ($race eq '7')
{
quest::MovePC(54, 10, -20, 0) ;
}
if ($race eq '130')
{
quest::MovePC(54, 10, -20, 0) ;
}
if ($race eq '330')
{
quest::MovePC(105, -18, -123, -16) ;
}
if ($race eq '5')
{
quest::MovePC(61, 94, -25, 3.75) ;
}
if ($race eq '12')
{
quest::MovePC(55, -35, -47, 4) ;
}
if ($race eq '1')
{
quest::MovePC(8, 211, -296, 4) ;
}
if ($race eq '11')
{
quest::MovePC(19, 45.3, 1.6, 3.8) ;
}
if ($race eq '3')
{
quest::MovePC(24, -309.75, 109.64, 23.75) ;
}
if ($race eq "Drakkin")
{
quest::MovePC(394, -550, -430, 80) ;
}
if ($race eq '128')
{
quest::MovePC(106, -416, 1343, 4) ;
}
if ($race eq '9')
{
quest::MovePC(52, 0, -100, 4) ;
}
if ($race eq '10')
{
quest::MovePC(37, -99, -345, 4) ;
}
if ($race eq '2')
{
quest::MovePC(29, 0, 0, 3.75) ;
}
if ($race eq '8')
{
quest::MovePC(60, -2, -18, 3.75) ;
}
}
}
|
 |
|
 |

01-11-2010, 06:35 PM
|
Sarnak
|
|
Join Date: Mar 2009
Location: none
Posts: 30
|
|
quest::movepc is case sensitive, stop camel-casing it.
|

01-11-2010, 07:30 PM
|
 |
Developer
|
|
Join Date: Aug 2006
Location: USA
Posts: 5,946
|
|
He is probably using an older version of GeorgeS' Quest Editor Tool. Until the most recent version of the tool, it was automatically changing the case of MovePC like that. Either upgrade the tool or edit the script from notepad/notepad++.
|
 |
|
 |

01-14-2010, 09:42 AM
|
Fire Beetle
|
|
Join Date: Nov 2009
Posts: 21
|
|
ah yes, i had the last version of georges tool and it was being crappy on me and changing the case, thanks trevius  . heres a working script for anyone that wants it
Code:
#Perl Script for Albert to teleport People to their respective starting cities
sub EVENT_SAY {
my $go = quest::saylink("go home");
if ($text =~/Hail/i) {
quest::say ("Good day to you, $name. I imagine you have talked to Death by now? If you haven't, you really should seek him out. If you have, are you ready to [$go]?");
}
if ($text =~/go home/i) {
if ($race eq 'Dark Elf') {
quest::movepc(40, 156.92, -2.94, 31.75) ;
}
if ($race eq 'Troll') {
quest::movepc(52, 0, -100, 4) ;
}
if ($race eq 'Wood Elf') {
quest::movepc(54, 10, -20, 0) ;
}
if ($race eq 'Half-Elf') {
quest::movepc(54, 10, -20, 0) ;
}
if ($race eq 'Vah Shir') {
quest::movepc(54, 10, -20, 0) ;
}
if ($race eq 'Froglok') {
quest::movepc(105, -18, -123, -16) ;
}
if ($race eq 'High Elf') {
quest::movepc(61, 94, -25, 3.75) ;
}
if ($race eq 'Gnome') {
quest::movepc(55, -35, -47, 4) ;
}
if ($race eq 'Human') {
quest::movepc(8, 211, -296, 4) ;
}
if ($race eq 'Halfling') {
quest::movepc(19, 45.3, 1.6, 3.8) ;
}
if ($race eq 'Erudite') {
quest::movepc(24, -309.75, 109.64, 23.75) ;
}
if ($race eq 'Drakkin') {
quest::movepc(394, -550, -430, 80) ;
}
if ($race eq 'Iksar') {
quest::movepc(106, -416, 1343, 4) ;
}
if ($race eq 'Troll') {
quest::movepc(52, 0, -100, 4) ;
}
if ($race eq 'Ogre') {
quest::movepc(37, -99, -345, 4) ;
}
if ($race eq 'Barbarian') {
quest::movepc(29, 0, 0, 3.75) ;
}
if ($race eq 'Dwarf') {
quest::movepc(60, -2, -18, 3.75) ;
}
}
}
|
 |
|
 |
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is Off
|
|
|
All times are GMT -4. The time now is 02:07 AM.
|
|
 |
|
 |
|
|
|
 |
|
 |
|
 |